はじめに
matplotlibのaxes.Axes.fill_betweenxにより、曲線で囲まれた範囲をx方向に塗りつぶす方法について説明する。
コード
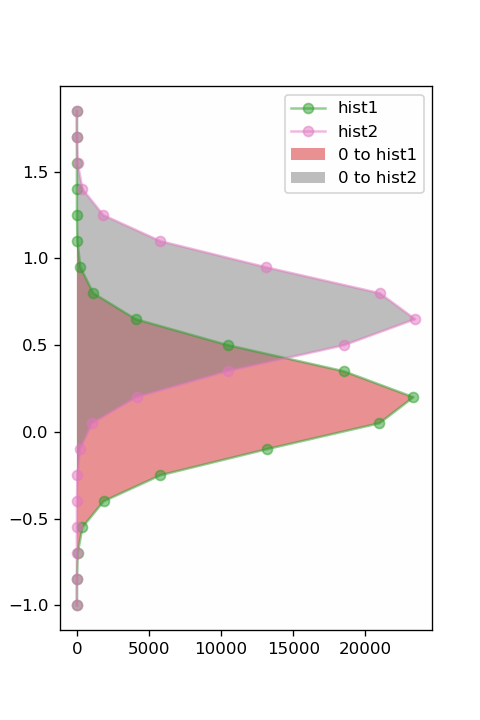
解説
モジュールのインポート
バージョン
データの生成
np.random.normal(mu, sigma, 100000)で平均mu,標準偏差sigmaのガウス分布に従うデータを100000点作成する。
np.histogram(d1, bins=20, range=(-1,2))でd1のデータのヒストグラムのデータ(binと頻度)をbin数20、-1から2の範囲で作成する。
binと頻度のサイズをbins1=bins1[:-1]により合わせている。
fill_betweenxによる塗りつぶし
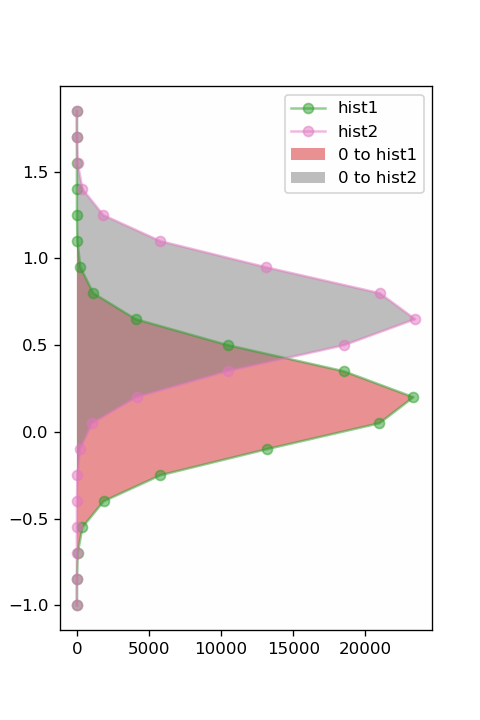
ax.fill_betweenx(bins1, 0, hist1)により、yがbins1の範囲でx=0からx=histの範囲を塗りつぶす。
whereによる塗りつぶす範囲の指定
where=(hist2 <= hist1)により、hist2がhist1以下となっている範囲のみを塗りつぶすことができる。
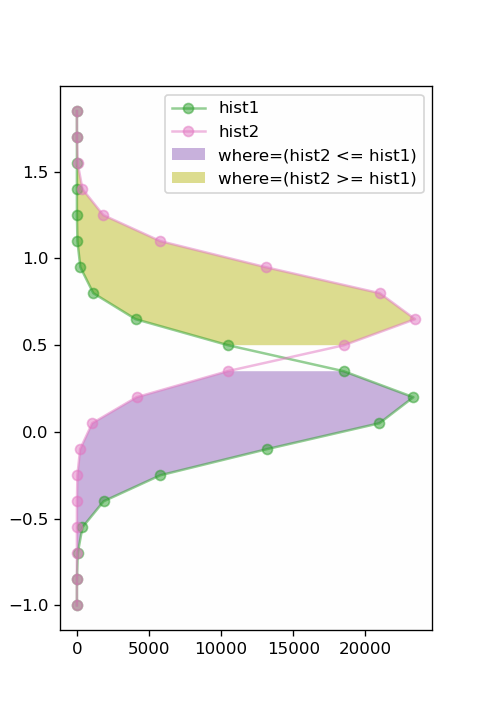
interpolate=Trueによる塗りつぶす範囲の補間
interpolate=Trueとすることで線が交差するところで生じている空白を塗りつぶすことができる。defaultではFalseとなっている。
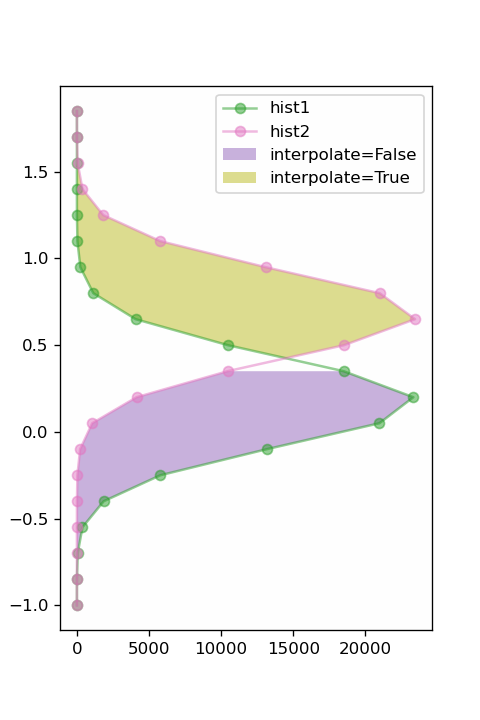
参考
matplotlib.axes.Axes.fill_betweenx — Matplotlib 3.3.1 documentation
numpy.random.normal — NumPy v1.26 Manual
numpy.histogram — NumPy v1.26 Manual
コメント