はじめに
skimage.filtersのエッジ検出フィルタには、sobel,prewitt, farid、scharrがある。これらのフィルタの回転不変性は、farid、scharr、sobel、prewittの順によかったので、各種フィルタを適用した画像の比較結果を示す。
解説
モジュールのインポートなど
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import numpy as np
import matplotlib.pyplot as plt
from skimage.filters import sobel,prewitt,farid,scharr
from skimage.draw import disk
from mpl_toolkits.axes_grid1.inset_locator import inset_axes
バージョン
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#version
import matplotlib
print(matplotlib.__version__)
3.3.4
import skimage
print(skimage.__version__)
0.18.1
print(np.__version__)
1.19.5
画像データの作成
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
img = np.zeros((256, 256), dtype=np.double)
rr, cc = disk((128,128), 100)
img[rr, cc] = 1
rr, cc = disk((128,128), 98)
img[rr, cc] = 0
skimageのdiskで円を作成することができる。ここでは、中心が(128,128)で半径が100の円を作成して、その部分を1として、中心が(128,128)で半径が98の円を作成してその部分を0とすることで円弧の画像を作成した。
作成した画像を表示すると以下のようになる。
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig, ax = plt.subplots(dpi=140)
im = ax.imshow(img,cmap="Spectral",origin="lower")
plt.colorbar(im)
ax.set_title("Test data")
ax.set(xlabel="x",ylabel="y")
plt.savefig("disk_perimeter.png",dpi=130)
plt.show()
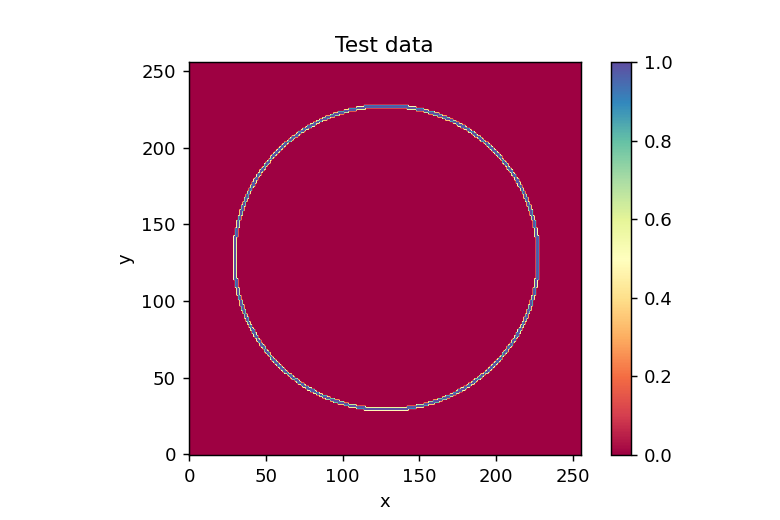
エッジフィルタの適用
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
so_data = sobel(img)
p_data = prewitt(img)
f_data = farid(img)
sc_data = scharr(img)
各種フィルタを画像中の各画素に適用することで画像の勾配を求めることができる。勾配が急激に変化するところがエッジとして検出される。各種フィルタは以下のようになる。
Prewittフィルタ
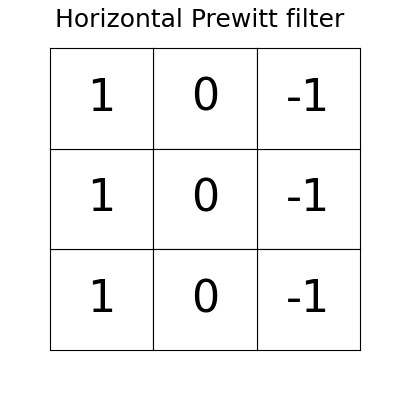
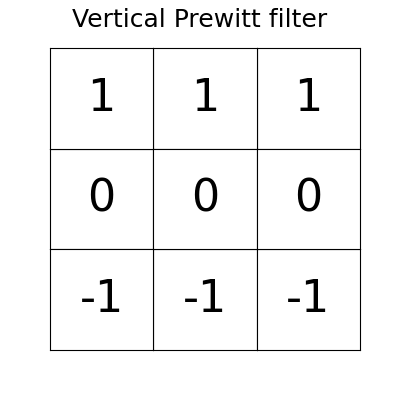
Sobelフィルタ
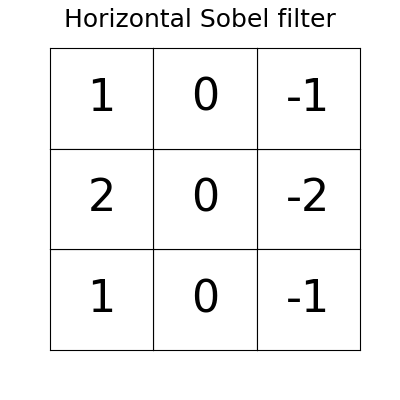
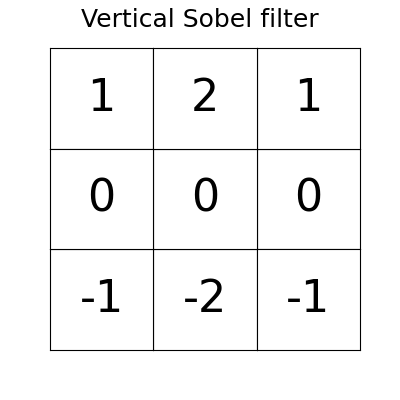
Scharrフィルタ
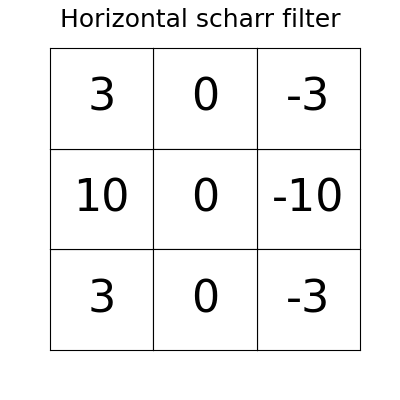
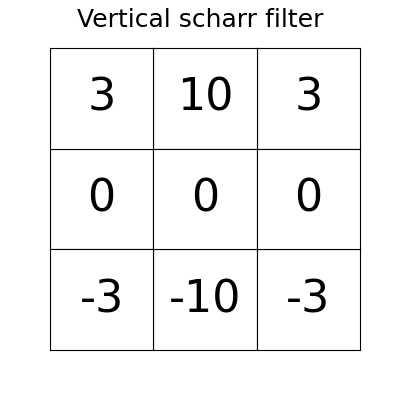
Faridフィルタ
Image derivative - Wikipedia
フィルタを適用した画像の表示
Prewittフィルタを適用した画像
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig, ax = plt.subplots(dpi=140)
im = ax.imshow(p_data,cmap="Spectral_r",origin="lower")
plt.colorbar(im)
ax.set_title("Prewitt filter")
ax.set(xlabel="x",ylabel="y")
plt.savefig("Prewitt.png",dpi=130)
plt.show()
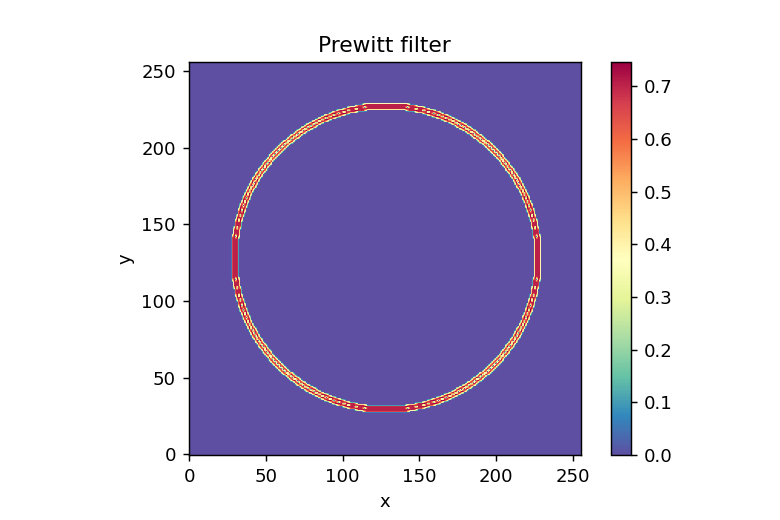
Sobelフィルタを適用した画像
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig, ax = plt.subplots(dpi=150)
im = ax.imshow(so_data,cmap="Spectral_r",origin="lower")
plt.colorbar(im)
ax.set_title("Sobel filter")
ax.set(xlabel="x",ylabel="y")
plt.savefig("Sobel.png",dpi=130)
plt.show()
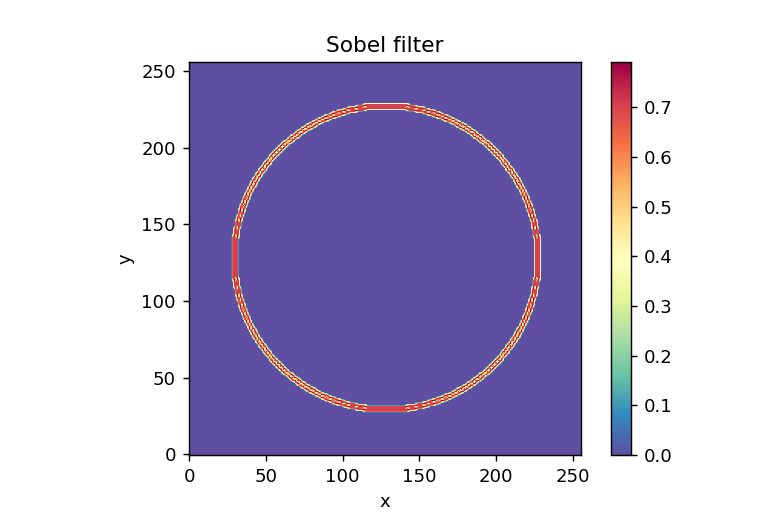
scharrフィルタを適用した画像
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig, ax = plt.subplots(dpi=140)
ax.imshow(sc_data,cmap="Spectral_r",origin="lower",vmin=0,vmax=1)
plt.colorbar(im)
ax.set_title("Scharr filter")
ax.set(xlabel="x",ylabel="y")
plt.savefig("Scharr.png",dpi=130)
plt.show()
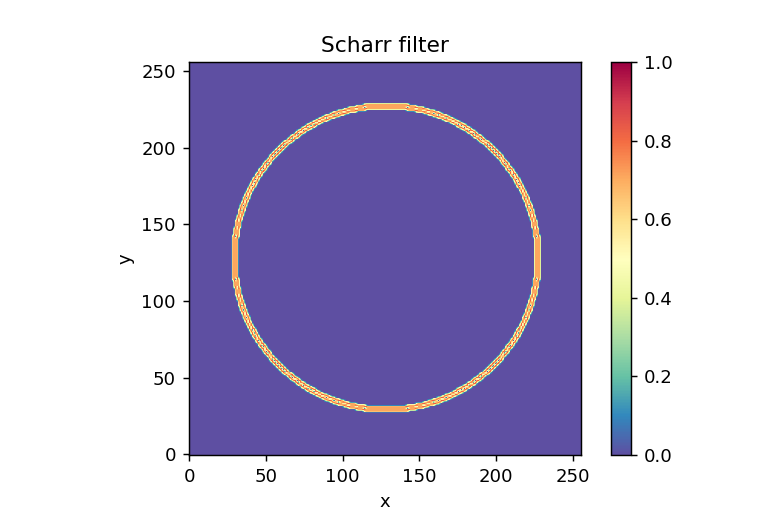
faridフィルタを適用した画像
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig, ax = plt.subplots(dpi=140)
im = ax.imshow(f_data,cmap="Spectral_r",origin="lower",vmin=0,vmax=1)
plt.colorbar(im)
ax.set_title("Farid filter")
ax.set(xlabel="x",ylabel="y")
plt.savefig("Farid.png",dpi=130)
plt.show()
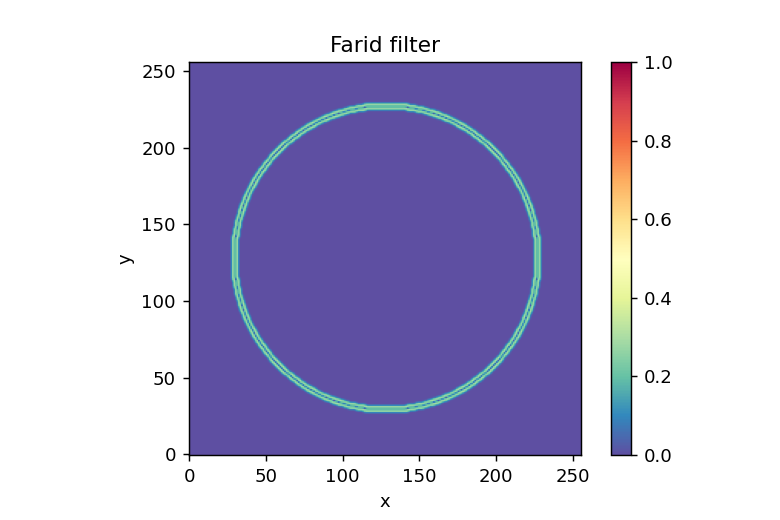
まとめて表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig, ax = plt.subplots(2,2,figsize=(6,6),dpi=140,sharex=True,sharey=True)
ax=ax.ravel()
im =ax[0].imshow(so_data,cmap="Spectral_r",origin="lower",vmin=0,vmax=1)
ax[1].imshow(p_data,cmap="Spectral_r",origin="lower",vmin=0,vmax=1)
ax[2].imshow(f_data,cmap="Spectral_r",origin="lower",vmin=0,vmax=1)
ax[3].imshow(sc_data,cmap="Spectral_r",origin="lower",vmin=0,vmax=1)
ax[0].set_title("Sobel filter")
ax[1].set_title("Prewitt filter")
ax[2].set_title("Farid filter")
ax[3].set_title("Scharr filter")
[ax[i].set(xlabel="x",ylabel="y") for i in range(4)]
axins = inset_axes(ax[3],
width="10%",
height="100%",
loc='lower left',
bbox_to_anchor=(1.05, -0.03, 1, 2.2),
bbox_transform=ax[3].transAxes,
)
fig.colorbar(im, cax=axins)
plt.savefig("edgefilter.png",dpi=130)
plt.show()
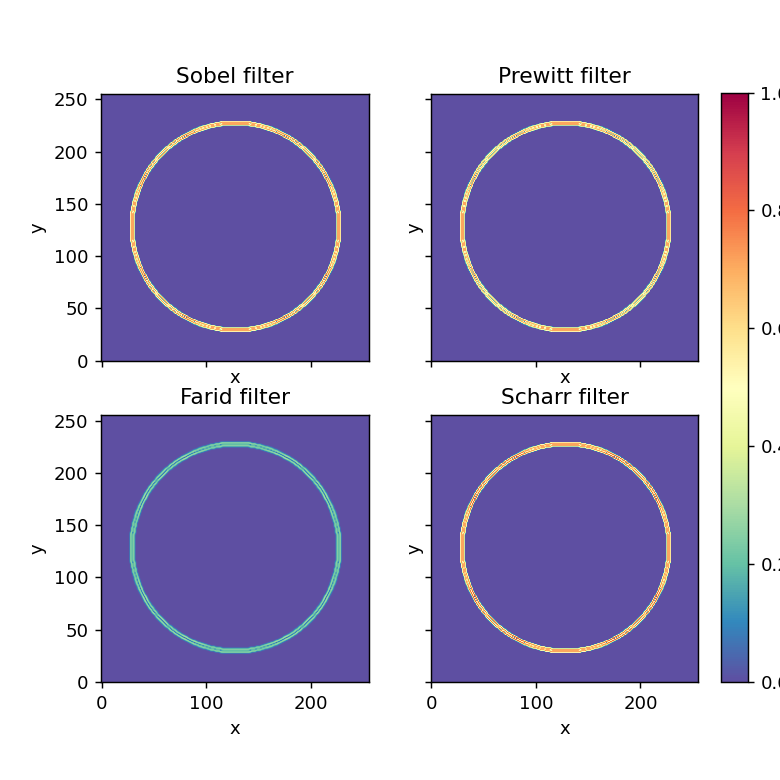
参考
skimage.filters — skimage 0.26.0rc0.dev0 documentation
skimage.filters — skimage 0.26.0rc0.dev0 documentation
skimage.filters — skimage 0.26.0rc0.dev0 documentation
skimage.filters — skimage 0.26.0rc0.dev0 documentation
コメント