はじめに
lmfitは非線形最小二乗法を用いてカーブフィットするためのライブラリであり、Scipy.optimize.curve_fitの拡張版に位置する。ここでは、lmfitでデータを指数関数的減衰モデルによりカーブフィッティングする方法について説明する。
コード
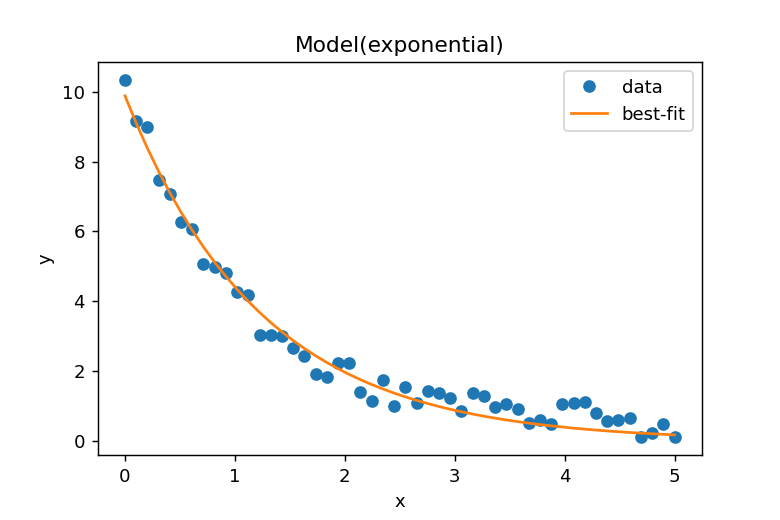
解説
モジュールのインポートなど
バージョン
データの生成
yデータはf(x,a,t)で定義した関数で作成する。rng = default_rng()とし、rng.random(50)でランダムデータを作成しyデータのノイズとする。xとyの関係を図で示すと以下のようになる。
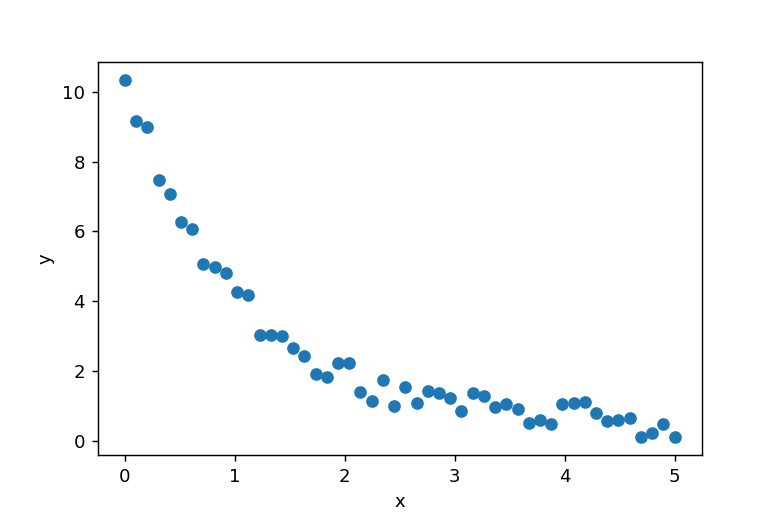
モデルの定義
lmfit.models
の ExponentialModel
をモデル関数として用いる。
初期パタメータの推定
model.guess(y, x=x)
により、上図のデータを指数関数的減衰関数で近似するためのフィッティングパラメータについて、初期値を推定する。パラメータ(params)は以下のようになる。
カーブフィット
model.fit(y, params, x=x)
により、カーブフィッティングを実行する。
フィッティング結果の表示
print(result.fit_report())
により、フィッティングの結果を見ることができる。
result.plot_fit()
によりデータとフィッティングカーブが表示される。
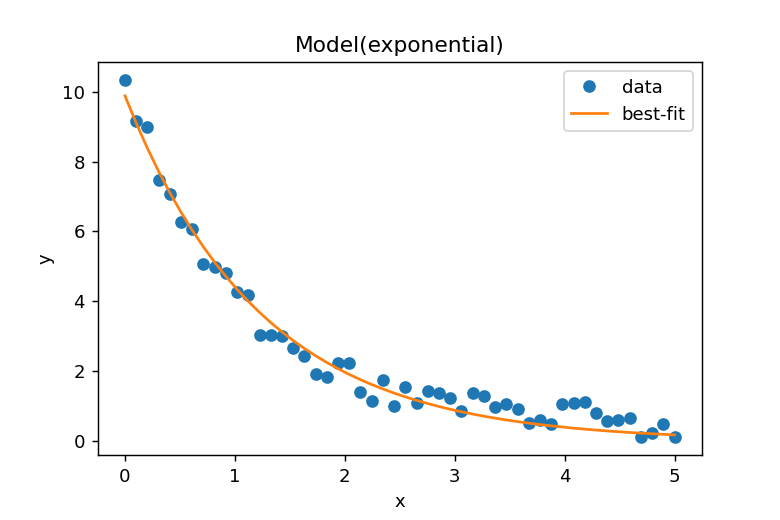
result.plot()
とすることで残差とともにフィッティング結果が表示される。
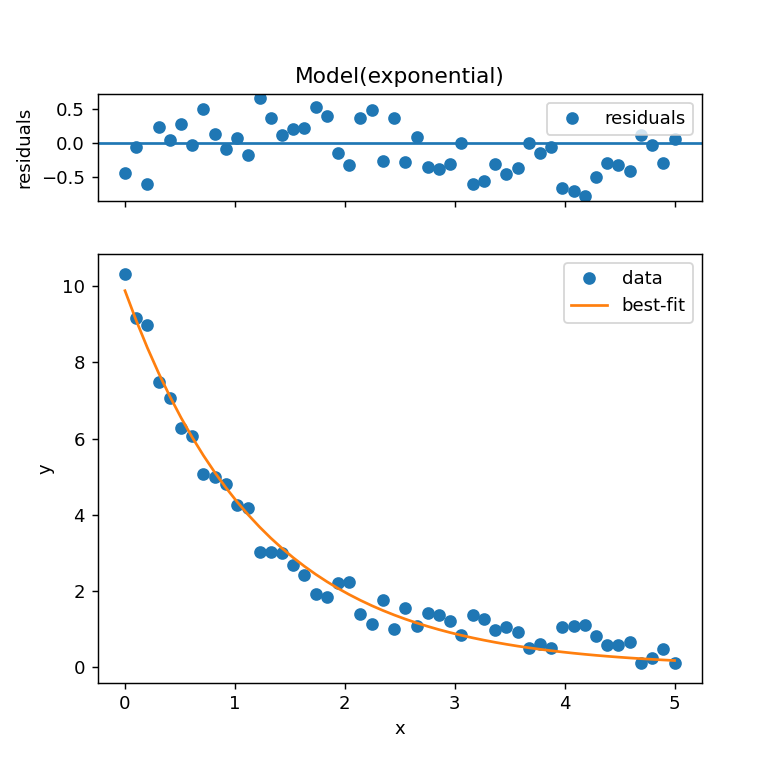
参考
Built-in Fitting Models in the models module — Non-Linear Least-Squares Minimization and Curve-Fitting for Python
Random Generator — NumPy v2.1 Manual
コメント