コード
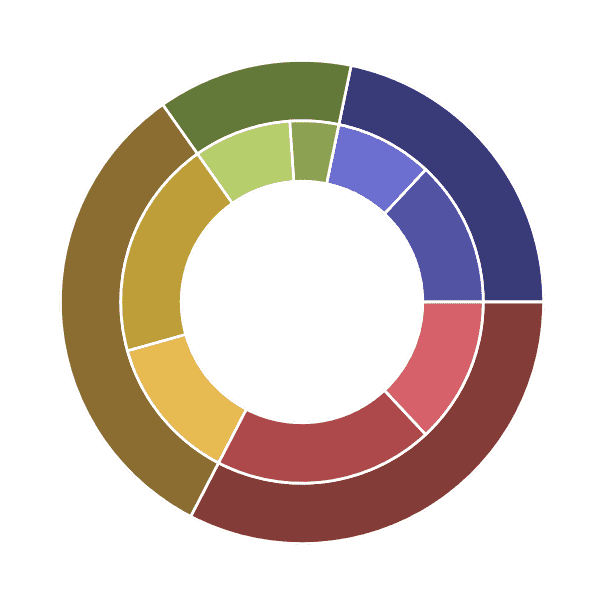
解説
はじめに
ax.pie()は円グラフを作成するメソッドだが、 円ではなくドーナツ状グラフもpie()メソッドで作成できる。 ここでは、2層構造をもつデータを、内側&外側のドーナツ状グラフで表示した例を説明する。 ドーナツ状のグラフを作成するには、wedgepropsの項目でwidthを設定すればいい。
モジュールのインポート
データの生成
(4, 2)の形状のてきとうなデータを生成。
色の設定
tab20bは、
https://matplotlib.org/examples/color/colormaps_reference.html
のQualitative colormapsにあり、同系色の色が4個ずつ連なったカラーマップとなっている。そのうち、外側のドーナツの色を0から4こ飛ばしで選択し、内側のドーナツの色を1,2個目、5, 6個目, 9, 10個目, 13,14個目と選択した。
ドーナツグラフの表示
vals.sum(axis=1)でnp.array全体ではなく、array内のarrayの合計を求めることができるので、4個のデータが得られる。
vals.flatten()は、np.arrayを1次元に展開するので、8個のデータが得られる。
colorsは、各要素に設定した各色が表示されることとなる。
sizeを0.25とし、width=sizeとすることでドーナツグラフとしている。
外側のグラフの半径を1とし、内側のグラフの半径を1-sizeとすることで、2層構造をうまく配置している。
参考
https://matplotlib.org/gallery/pie_and_polar_charts/nested_pie.html
https://matplotlib.org/api/_as_gen/matplotlib.axes.Axes.pie.html
コメント