はじめに
matplotlibのplt.barで表示する棒グラフの棒の色をグラデーションカラーで表示する方法について説明する。
コード
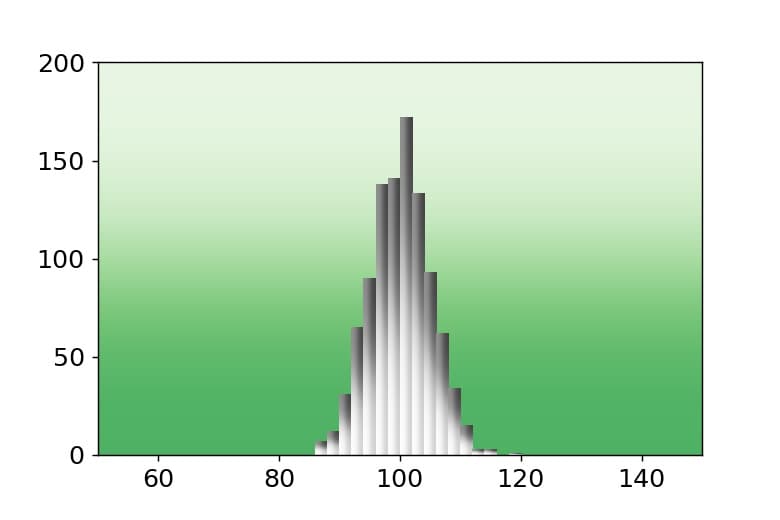
解説
モジュールのインポートなど
グラデーションカラー生成関数
axではグラデーションカラーを表示する図を指定する。
extentは図中の表示範囲の設定であり、デフォルトはデータ座標なので相対的な座標で設定するには、transform=ax.transAxesなどのようにする。
directionはグラデーションの方向であり、0で垂直方向、1で水平方向となる。
cmap_rangeではカラーマップの範囲を指定する。最低と最高はそれぞれ0と1となる。
phiで角度を指定して、単位ベクトルvでグラデーションの方向を定義する。Xで4隅の座標を得て、a,bの大きさに揃えたXを最終的に得る。これをinterpolation=’gaussian’でimshowすることでグラデーションカラーが得られる。
以下にdirectionを変化させたときの値の変化を示す。
#direction = 0.5
#direction = 1
#direction = 0
#direction = 0, a,b =.8,.2
a, bを降順にすることでグラデーションを逆にすることができる。
グラデーション棒グラフ生成関数
棒グラフの角棒のleft, right, bottom, topを取得して、gradient_image
を適用する。
軸範囲の設定
図の生成
背景の設定
extent=(0, 1, 0, 1), transform=ax.transAxesとすることで図全体にグラデーションカラーを表示できる。
データの生成
norm.rvsで正規分布に従うランダムデータを生成する。
棒グラフの表示
gradient_bar
でグラデーションカラー棒グラフを表示できる。
参考
Bar chart with gradients — Matplotlib 3.1.2 documentation
Interpolations for imshow — Matplotlib 3.9.2 documentation
matplotlib.pyplot.bar — Matplotlib 3.9.2 documentation
コメント
[…] [matplotlib] 58. 棒グラフのbarをグラデーションカラーで表示matplotlibのplt.barで表示する棒グラフの棒の色をグラデーションカラーで表示する方法について説明する。sabopy.com2020.01.29 […]