はじめに
簡単かつ簡潔にデータを可視化できるseabornを使って、各カテゴリー内のデータの分布をバイオリンプロット(violinplot)で表示する方法について説明する。
コード
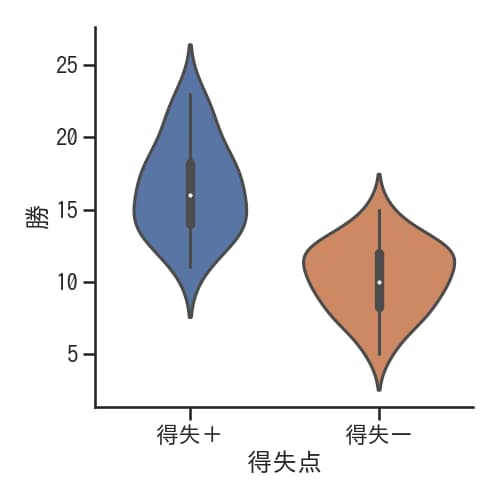
解説
モジュールのインポートなど
データの読み込み
データは下記サイトから2017〜2019シーズンのJ1の結果を取得し、pandasのDataFrameとした。
作成したDataFrameはpd.concatで結合した。
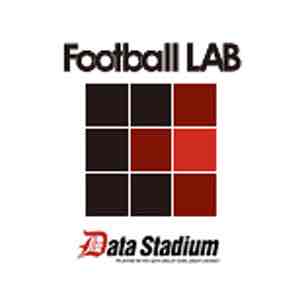
リーグサマリー:2019 J1 順位表 | データによってサッカーはもっと輝く | Football LAB
フットボールラボ(Football LAB)はサッカーをデータで分析し、新しいサッカーの観戦方法を伝えるサッカー情報サイトです。選手のプレーを評価するチャンスビルディングポイントやプレースタイル指標、チームの戦術を評価するチームスタイル指標...
新たな列データをDataFrameへ追加
得失のデータを使って得失点差がプラスなチームとマイナスなチームに分ける。プラスのチームには”得失+”をいれ、マイナスのチームには”得失ー”を入れる。
さらに、ランクについても同様に処理する。
DataFrameの最初の5行は以下のようになる。
順位 | Unnamed: 1 | Unnamed: 2 | 勝点 | 試合数 | 勝 | 分 | 敗 | 得点 | 失点 | 得失 | 平均得点 | 平均失点 | 得失点 | ランク | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | NaN | 横浜F・マリノス横浜FM | 70 | 34 | 22 | 4 | 8 | 68 | 38 | 30 | 2.0 | 1.1 | 得失+ | 上位 |
1 | 2 | NaN | FC東京FC東京 | 64 | 34 | 19 | 7 | 8 | 46 | 29 | 17 | 1.4 | 0.9 | 得失+ | 上位 |
2 | 3 | NaN | 鹿島アントラーズ鹿島 | 63 | 34 | 18 | 9 | 7 | 54 | 30 | 24 | 1.6 | 0.9 | 得失+ | 上位 |
3 | 4 | NaN | 川崎フロンターレ川崎F | 60 | 34 | 16 | 12 | 6 | 57 | 34 | 23 | 1.7 | 1.0 | 得失+ | 上位 |
4 | 5 | NaN | セレッソ大阪C大阪 | 59 | 34 | 18 | 5 | 11 | 39 | 25 | 14 | 1.1 | 0.7 | 得失+ | 上位 |
violinplotの表示
sns.catplot(x=”得失点”, y=”勝”, kind=”violin”,data=df)により、DataFrame(df)の得失点のカテゴリー別に勝データのviolinplotを表示する。
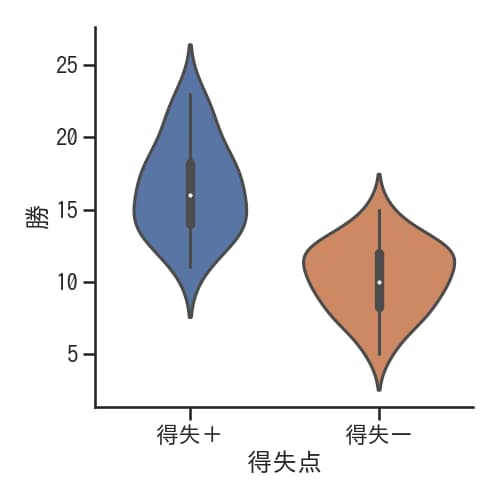
ヴァイオリン内部を変える
innerの値を変えることでviolin内部の表示を変えることができる。以下の4種類がある。
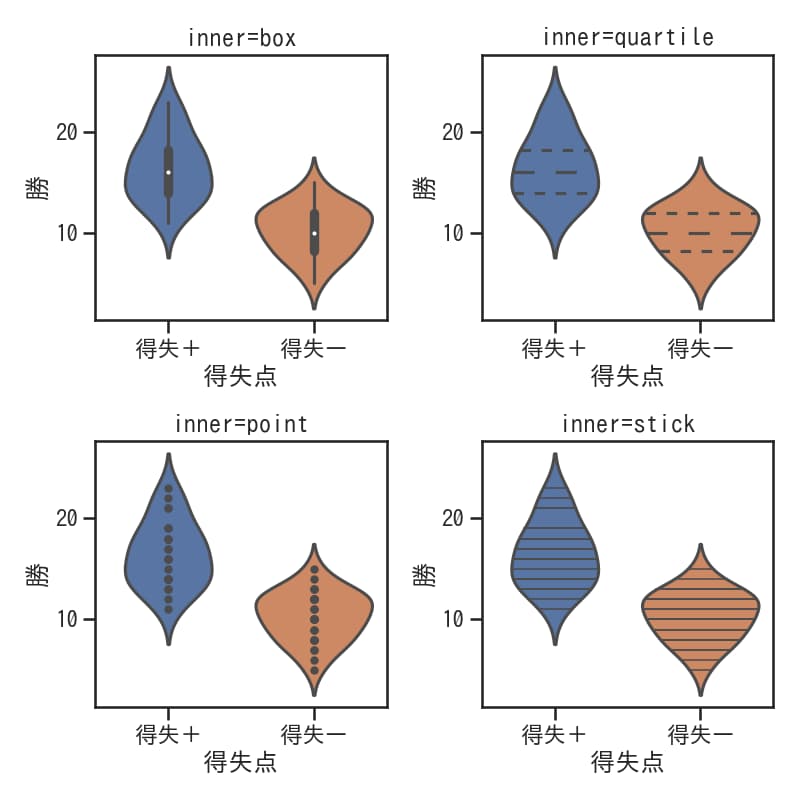
hueを設定した場合
hue=’ランク’とすることでランクに応じて色分けされたviolinplotが表示される。
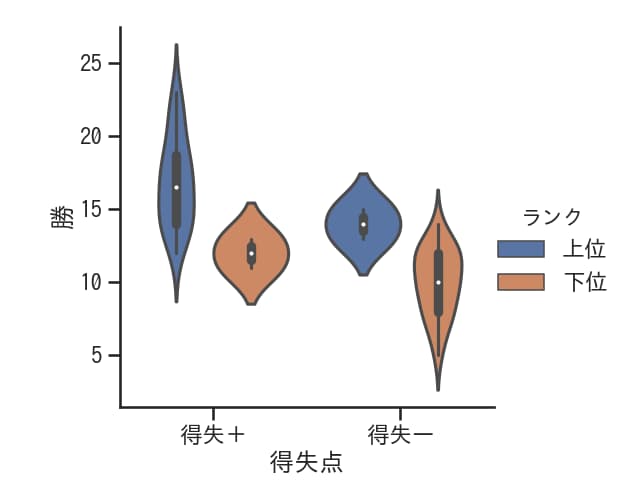
split=Trueでヴァイオリンの片側のみを表示
hueを設定した状態でsplit=Trueとすると片側のみにヴァイオリンを表示できる。
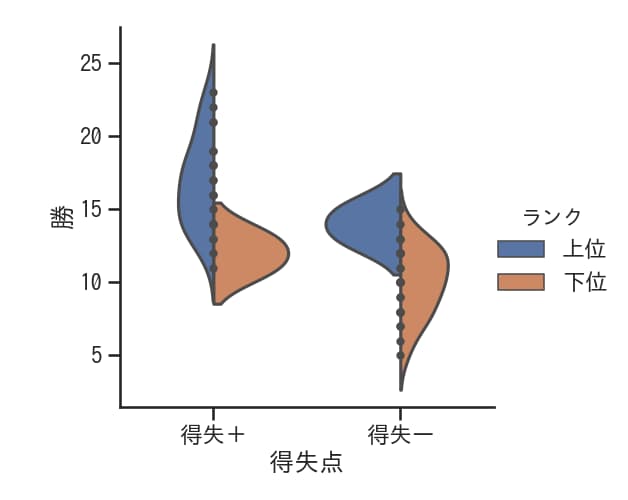
violinplotとswarmplotを合わせて表示
violinplot とswarmplotを組み合わせて表示することをできる。
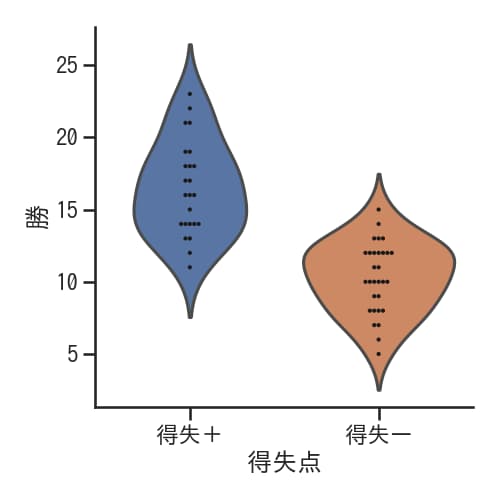
swarmplotについては下記で解説した。
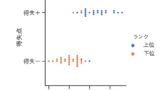
[seaborn] 8. stripplotとswarmplotで各カテゴリーのデータを散布図で表示
簡単かつ簡潔にデータを可視化できるライブラリであるseabornのstripplotとswarmplotを用いて、各カテゴリーのデータをそれぞれ散布図で表示する方法について説明する。
参考
Visualizing categorical data — seaborn 0.13.2 documentation
seaborn.violinplot — seaborn 0.13.2 documentation
コメント