はじめに
scipyのndimageのgaussian_laplaceを使って、LoGフィルタで画像のエッジを検出して表示する方法について説明する。
コード&解説
モジュールのインポート
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import matplotlib.pyplot as plt
import numpy as np
from skimage.color import rgb2gray
import scipy.ndimage as ndi
plt.rcParams['figure.dpi'] = 150
バージョン
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#version
import matplotlib,skimage,scipy
print(skimage.__version__)
print(matplotlib.__version__)
print(scipy.__version__)
print(np.__version__)
0.19.2
3.5.1
1.8.0
1.22.3
画像の読み込み
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#load sample dataset
img = plt.imread("taiyaki_tennen_in.jpg")#https://www.irasutoya.com/2022/04/blog-post_11.html
下記サイトから画像を取得し、plt.imread()で読み込む。
たい焼き器のイラスト(天然)
いらすとやは季節のイベント・動物・子供などのかわいいイラストが沢山見つかるフリー素材サイトです。
グレースケール変換
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
img_g = rgb2gray(img)
skimage.color の rgb2grayでRGB画像をグレースケール画像に変換する。
変換した画像をcmap=”bone”で表示すると以下のようになる。
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#show
fig,ax=plt.subplots()
ax.imshow(img_g,cmap="bone")
ax.axis("off")
plt.savefig("LoG_1.jpg",dpi=150)
plt.show()
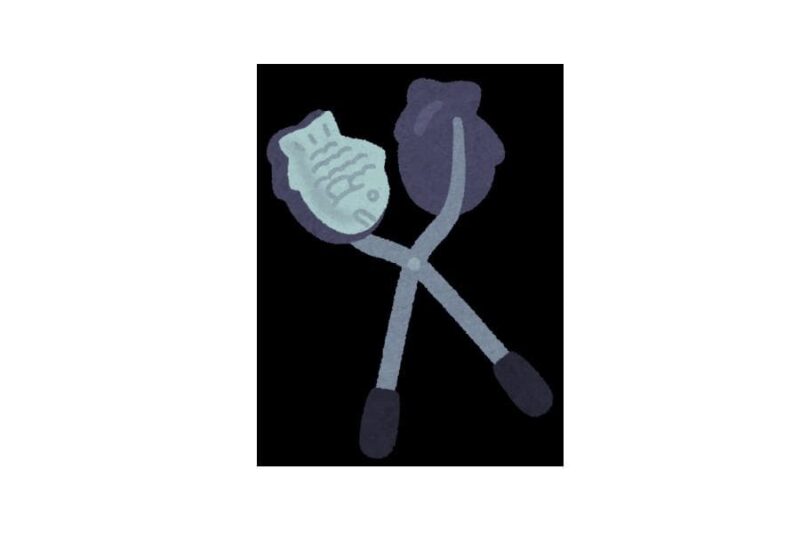
LoGフィルタ
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#gaussian_laplace
result = ndi.gaussian_laplace(img_g, sigma=3)
fig,ax=plt.subplots(1,2,figsize=(8,4))
ax=ax.ravel()
ax[0].imshow(img_g,cmap="bone")
im=ax[1].imshow(result,cmap="viridis")
plt.colorbar(im)
for a in ax:
a.axis("off")
ax[0].set_title("Original")
ax[1].set_title("gaussian_laplace")
plt.savefig("LoG_2.jpg",dpi=100)
plt.show()
LoGフィルタであるgaussian_laplaceをsigma=3として用いることで、以下のようになる。
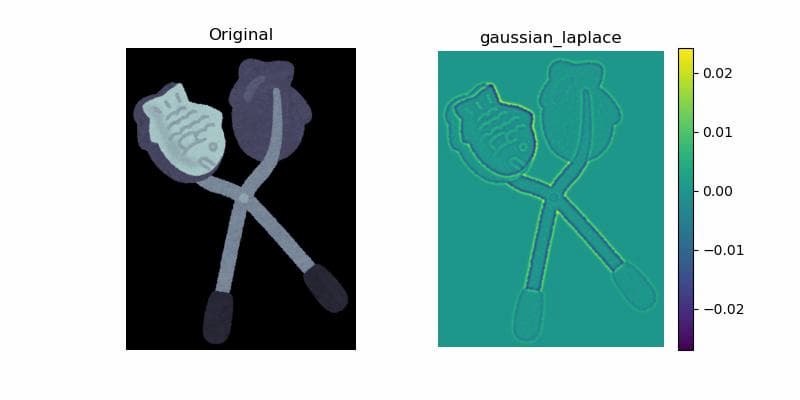
手動でLoGフィルタ
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#manually gaussian_laplace
sigma = 3
im_x =ndi.gaussian_filter(img_g, (sigma,sigma), (0,2))
im_y =ndi.gaussian_filter(img_g, (sigma,sigma), (2,0))
magnitude = im_x+im_y
fig,ax=plt.subplots(1,3,figsize=(8,3.5))
ax=ax.ravel()
ax[0].imshow(im_x)
ax[1].imshow(im_y)
ax[2].imshow(magnitude,cmap="viridis")
for a in ax:
a.axis("off")
#ax.set_title('filt="gaussian"')
ax[0].set_title("dx$^2$")
ax[1].set_title("dy$^2$")
ax[2].set_title("gaussian_laplace")
plt.savefig("LoG_3.jpg",dpi=100)
plt.show()
LoGフィルタはガウス関数の2階微分フィルタであるため、x方向の結果は結果は、ndi.gaussian_filter(img_g, (sigma,sigma), (0,2))で得られる。yはorderを(2,0)とした場合に得られる。強度の像は各成分の和として得られる。
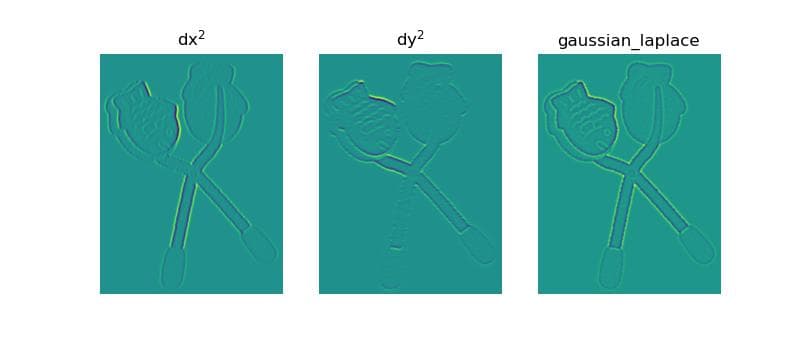
LoGフィルタのsigmaを変えた場合
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#gaussian_laplace sigma
ggms = []
sigmas=[1,3,5,7]
for i in sigmas:
result = ndi.gaussian_laplace(img_g, sigma=i)
ggms.append(result)
fig,ax=plt.subplots(2,2,figsize=(6,7))
ax=ax.ravel()
[ax[i].imshow(ggms[i],cmap="viridis") for i in range(len(ggms))]
[ax[i].set_title("sigma="+str(sigmas[i])+"") for i in range(len(ggms))]
for a in ax:
a.axis("off")
plt.suptitle('gaussian_laplace')
plt.savefig("LoG_4.jpg",dpi=100)
plt.show()
ガウス微分フィルタのsigmaを変えた時の変化は以下のようになる。
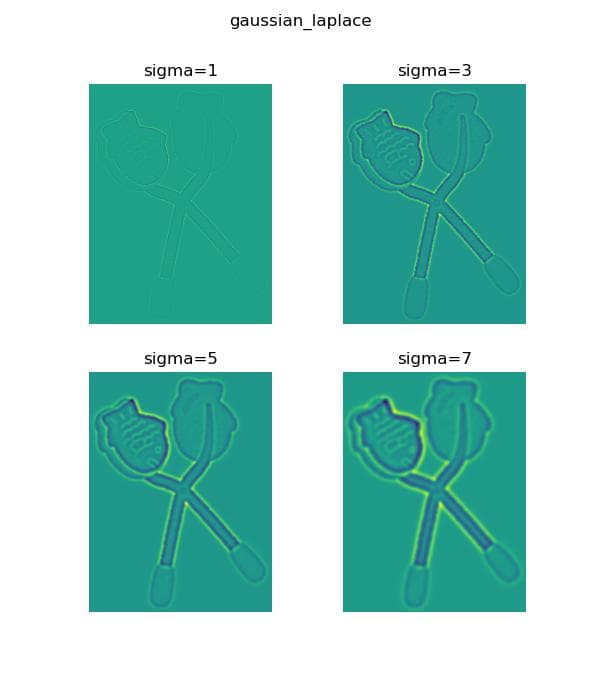
参考
gaussian_laplace — SciPy v1.15.2 Manual
gaussian_filter — SciPy v1.15.2 Manual
コメント