はじめに
Voxelグラフで作成したNumPyロゴの色を変化させたアニメーションをmatplotlib FuncAnimationで表示する。
コード
解説
モジュールのインポート
3Dグラフの作成
3Dグラフとするために、ax を fig.gca(projection=’3d’)とする。
Numpyロゴの設定
データの生成
n_voxels = np.zeros((4, 3, 4), dtype=bool)では、bool値は0ならFalse、0以外ならTrueとなる。つまり、n_voxelsは4×3×4のFalseの配列となる。
n_voxels[0, 0, :] = Trueでn_voxelsの[0, 0, :]の部分がTrueになる。一連のTrue化により、Nの部分をTrueとしている。
facecolor, edgecolorの初期設定
n_voxelsのTrueの部分を’k'(黒)として、Falseの部分を’k'(黒)とする。facecolorはアニメーションで変化させるので最初は両方とも黒にしている。edgecolorはすべてのボクセルで黒とした。
データの拡張
fillledはn_voxelsと同じかたちの要素がすべて1の配列。
filled_2はfilledをに下のexplode()関数を適用したものとなる。
dataの要素の2倍の大きさを要素として持つsizeという配列を作り、sizeの各配列の大きさを1小さくした0の配列(data_e)をつくって、data_eに2こ飛ばしでdataの要素を入れていくことになる。
ボクセル間の隙間の設定
(filled_2.shape) + 1の形状の、要素として各配列のindexをもつ配列を作成して、その値を2でわって切り捨てにした要素をもつ配列をつくる。
各要素の+0.05などをして、うまいこと隙間を開けていく。
アニメーションの設定
ここでは、カラーサイクルのリストから色をランダムに選択する手法をとる。
カラーサイクルとは、複数のデータをプロットした時に自動的に設定される色のことで、 plt.rcParams[‘axes.prop_cycle’].by_key()[‘color’]とすることでリスト形式で取り出すことができる。
このリストの中からひとつをnp.random.choice(cycle)とすることで取り出し、col1, col2を設定する。そして、col1, col2をnp.where()により、TrueとFalseの位置にそれぞれ設定する。
ax.voxels(x, y, z, filled_2, facecolors=fcolors_2, edgecolors=ecolors_2)でボクセルグラフを表示する。x,y,zがボクセルの左手前下の座標、filled_2で色をつける位置を指定している。
アニメーションの表示
25stepアニメーション関数を実行して、200 ms間隔で順次図をかえていくので、5 secのアニメーションとなる。
HTML(ani.to_html5_video())とすればjupyter notebook上にアニメーションを表示できる。
参考
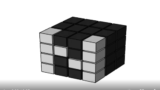
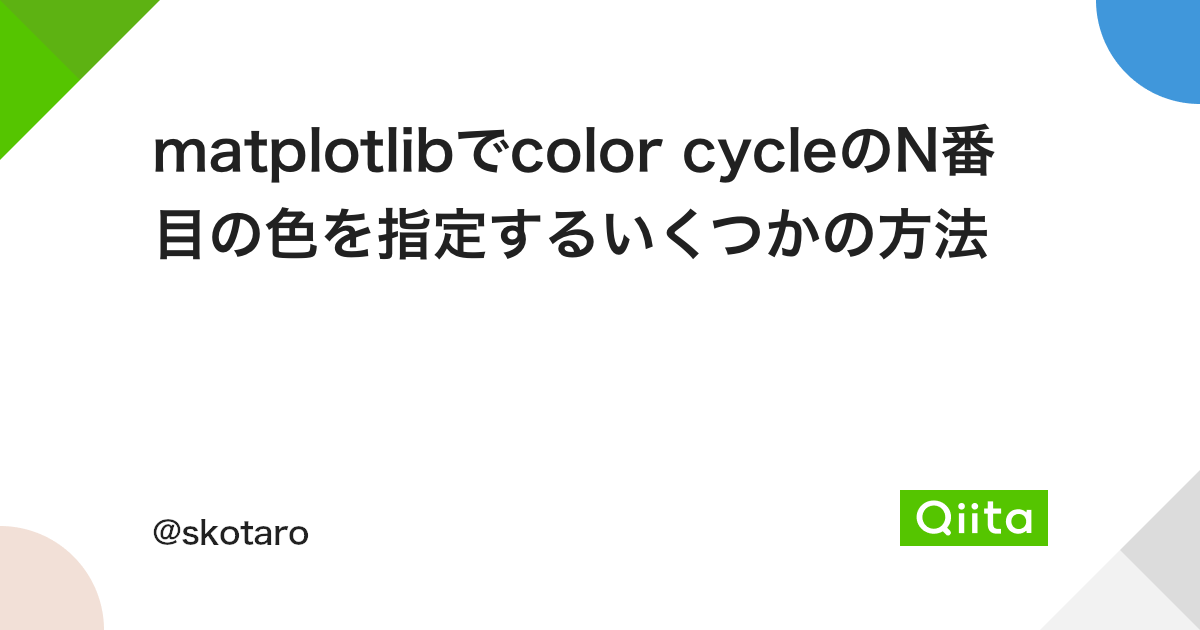
コメント