はじめに
matplotlib.datesのConciseDateFormatterで時系列グラフの目盛りラベルを簡潔に表示する方法について説明する。
コード
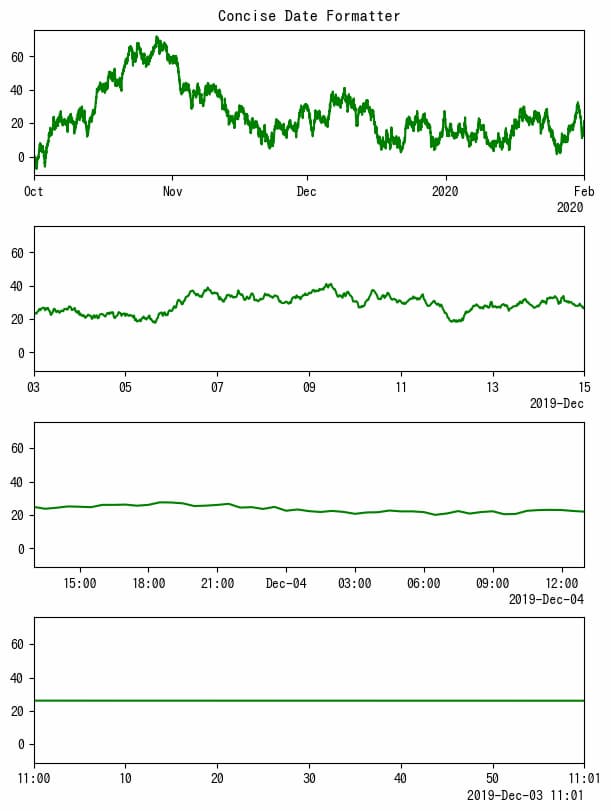
解説
モジュールのインポート
バージョン
データの生成
dates = np.array([base + datetime.timedelta(hours=(0.5*i)) for i in range(24*256)])で baseの日付から、0.5h刻みで24 ✕256 = 6144回時間を増加させた配列が得られる。
表示範囲の設定
デフォルト設定の時系列グラフ
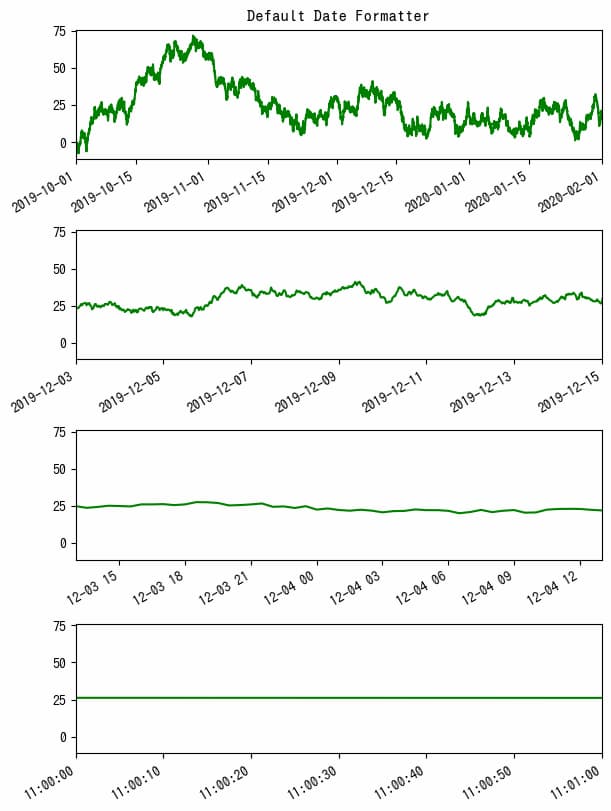
時間の表示範囲によって目盛りラベルが変化するが基本的にどれも長いので、重なりを防ぐ必要がある。ここでは、label.set_rotation(30)で30°回転している。
ConciseDateFormatterを使った時系列グラフ
最初に、locator = mdates.AutoDateLocator(minticks=4, maxticks=9)
で目盛りの数などを決め、formatter = mdates.ConciseDateFormatter(locator)
により、ConciseDateFormatter
をlocato
rに適用する。
グラフに設定を反映させるには、ax.xaxis.set_major_locator(locator)
とax.xaxis.set_major_formatter(formatter)
とする必要がある。
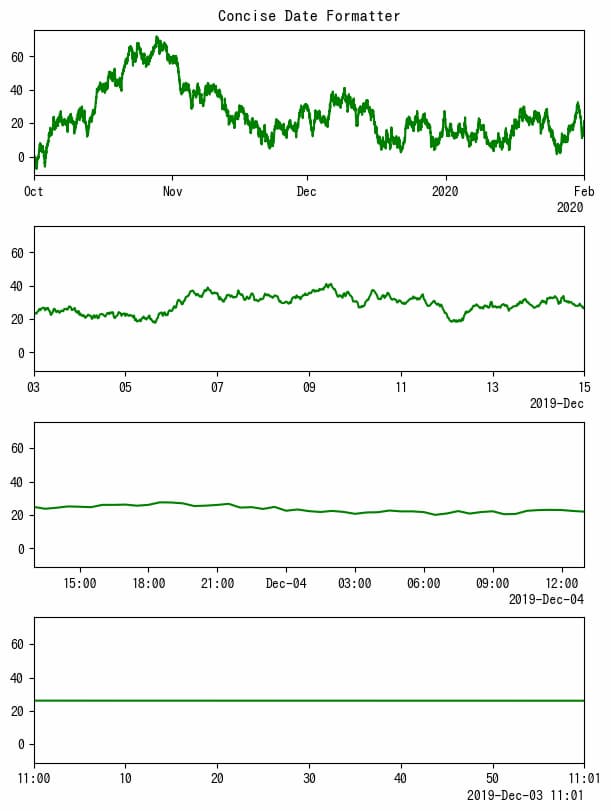
units registryを使ってConciseDateFormatterを適用させる方法
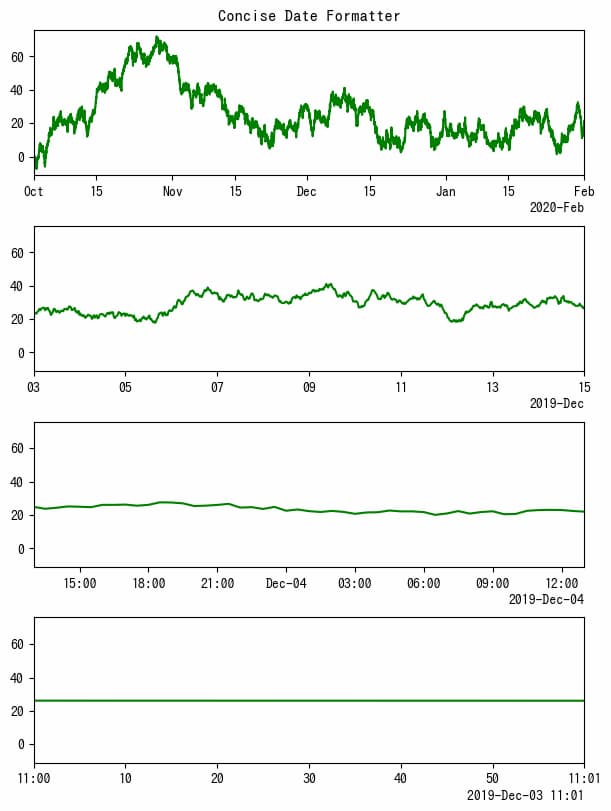
converter = mdates.ConciseDateConverter()
とし、munits.registry[np.datetime64] = converter
としておけば、set_major_formatter
などをする必要がないので、より簡単に軸ラベルを簡素化できる。
参考
Formatting date ticks using ConciseDateFormatter — Matplotlib 3.4.3 documentation
コメント