はじめに
skimage.measureのfind_contoursで、バイナリ画像上で対象物の輪郭を検出して画像中に表示する方法について説明する。
コード
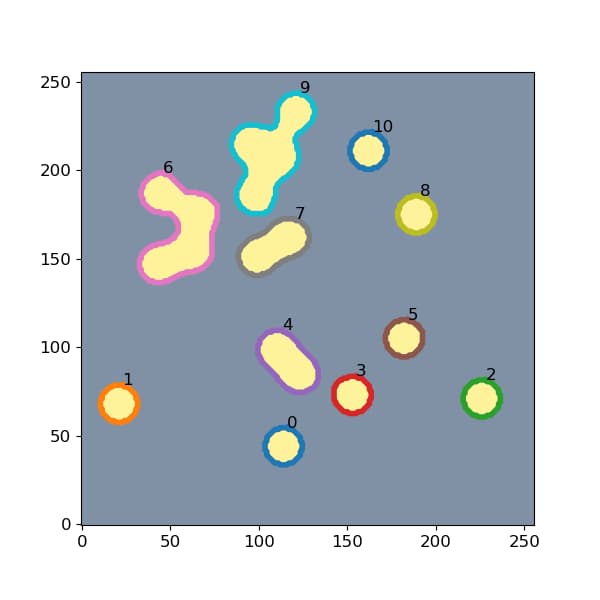
解説
モジュールのインポートなど
画像の生成
最初にnp.zeros((s, s))
で256 x 256のデータを生成する。
次に、(s*np.random.random((2, n**2))).astype(int)
で0〜255のランダムな整数を(2,36)の形状で生成する。そして、imの[points[0], points[1]]に該当する部分の要素を1として、ndimage.gaussian_filterでぼかすことで画像データを生成した。
threshold_otsuで大津の方法によるしきい値を取得する。
このしきい値で画像を2値化し、境界に接している部分をclear_borderで除去する。
輪郭を検出
find_contoursで輪郭を検出する。
バイナリ画像の場合、検出に用いる値は0と1の中間の0.5とする。
結果の表示
figを作成した後に、coutoursの中に格納されている輪郭データをenumerateを用いて一つずつプロットしていく。データの開始点の座標にax.text()でインデックスを表示する。
ax.imshowで画像を表示すれば、輪郭に線を描写した画像が得られる。
find_contoursの値を変えたときの変化
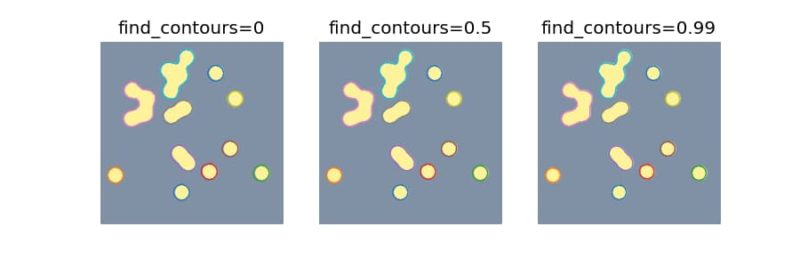
左の図から順にfind_contoursの値を0,0.5,0.99としたときの結果を示した。見た目の変化は見られないが、輪郭データの配列の形状を比較すると以下のようになる。
0でfind_contours したcontours0のみ要素数が少ないことがわかる。
さらに0.99と0.5でfind_contoursしたcontours1とcontours05の差を取ると以下のようになる。値が僅かに異なっていることがわかる。
コードをダウンロード(.pyファイル)
コードをダウンロード(.ipynbファイル)
参考
skimage.measure — skimage 0.23.3rc0.dev0 documentation
コメント