はじめに
ipywidgetsのColorPickerで色を選択し、整列した無着色のマーカーをクリックして着色する方法について説明する。また、色の集計を行い棒グラフで表示する。
コード
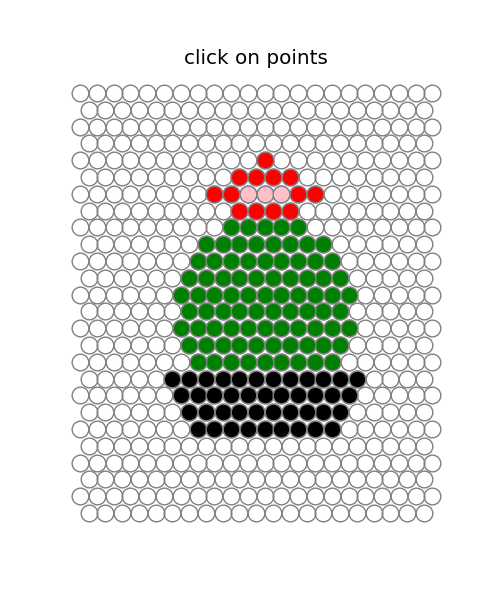
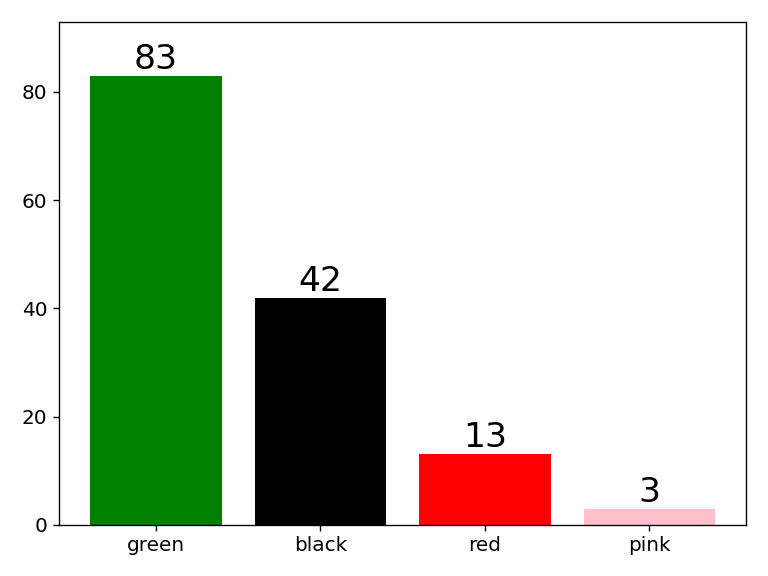
解説
モジュールのインポートなど
%matplotlibでjupyter lab, notebook内ではなく、別ウインドウでQt5Aggのbackendにより表示する。
バージョン
データの生成
np.meshgridでgrid状のデータを作成する。図示すると以下のようになる。
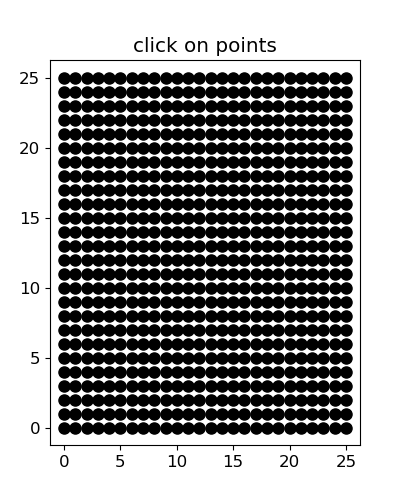
データの加工
xの1以下、24以上をnp.nanとする。さらにxの偶数行のインデックスが2の位置をnp.nanとし、-0.5することでマーカーをずらす。
図の表示
ColorPickerの表示
ColorPickerでは色のついた四角をクリックすることで出てくるカラーピッカーウインドウから色を選択できる。また、pinkなどの色の名前を直接入力することでも色を選択できる。
onpickイベントの設定
最初にマーカーの色を入れるための空のリスト(mfcs)を作成しておく。
event.artistをthislineとして、get_xdata()、get_ydata()でクリックした点のdataの配列を取得し、event.indでインデックスを取得することで、クリックした点の座標を取得する。
ax.plot()でmfc=”+colp.value+”とすることでカラーピッカーで選択した色の点がクリックしたマーカーに表示される。
plot.get_markerfacecolor()でマーカーの色を取得し、リストにいれる。このリストは後で集計するために用いる。
fig.canvas.draw()で図を更新する。
fig.canvas.mpl_connect(‘pick_event’, onpick)でイベントを実行可能とする。
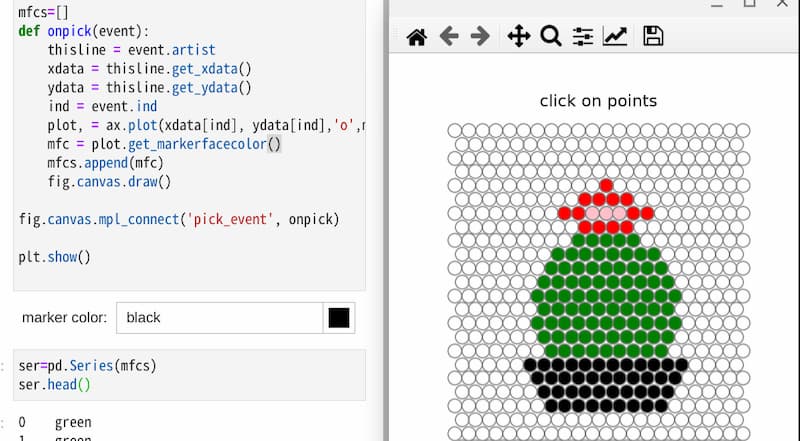
色の集計
pd.Series()でlistをpandasのシリーズとし、シリーズに対して.value_counts()を行うことで要素のカウントを行った。
集計結果の表示
plt.bar()で棒グラフを表示する。color=counts.indexとすることでインデックスの色を棒グラフの色とした。
plt.text()で値をそれぞれの棒の上に表示した。
コメント