はじめに
lmfitは非線形最小二乗法を用いてカーブフィットするためのライブラリであり、Scipy.optimize.curve_fitの拡張版に位置する。ここでは、データを重み付きガウス分布関数モデルによりカーブフィッティングする方法について説明する。
コード
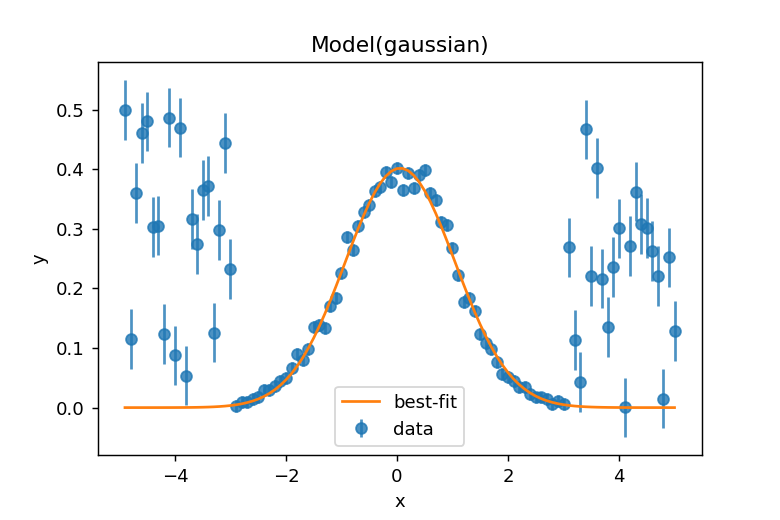
解説
モジュールのインポート
バージョン
データの生成
rng = default_rng()とし、rng.normal(0,1,10000)で標準正規分布に従うランダムデータを10000こ作成する。xとyの関係を図で示すと以下のようになる。
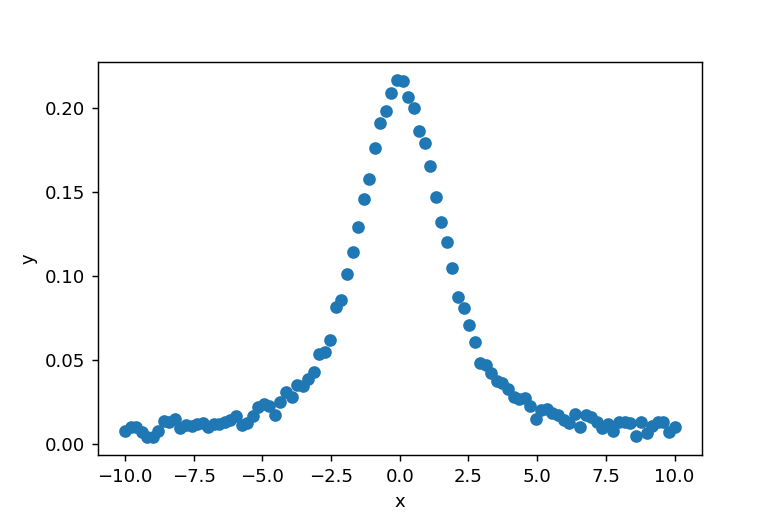
y値に両端の20こ目までランダムなデータを付加する。xとyの関係を図で示すと以下のようになる。
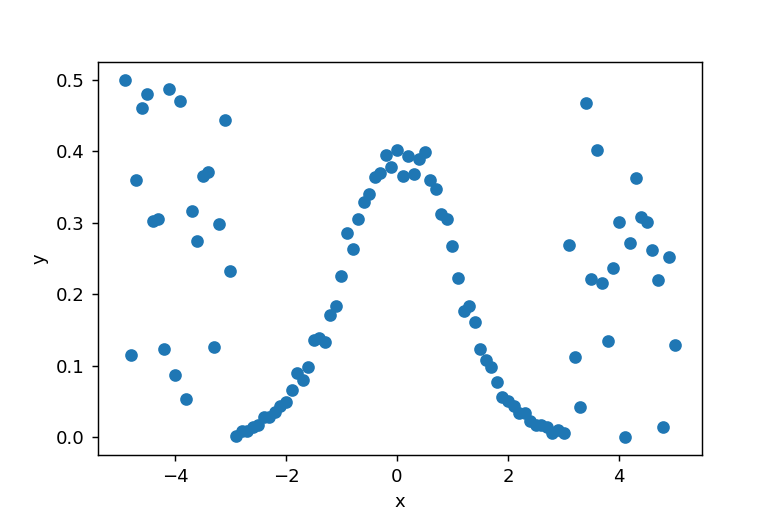
モデルの定義
lmfit.models
の GaussianModel
をモデル関数として用いる。Gaussian関数は以下の式で表される。パラメータはamplitude, center, sigmaとなる。
\[f(x; A, \mu, \sigma) = \frac{A}{\sigma\sqrt{2\pi}} e^{[{-{(x-\mu)^2}/{{2\sigma}^2}}]}\]
初期パタメータの推定
model.guess(y, x=x)
により、上図のデータをガウス関数モデルで近似するためのフィッティングパラメータについて、初期値を推定する。パラメータ(params)は以下のようになる。
カーブフィット
model.fit(y, params, x=x)
により、カーブフィッティングを実行する。
フィッティング結果の表示
print(result.fit_report())
により、フィッティングの結果を見ることができる。
result.plot_fit()
によりデータとフィッティングカーブが表示される。
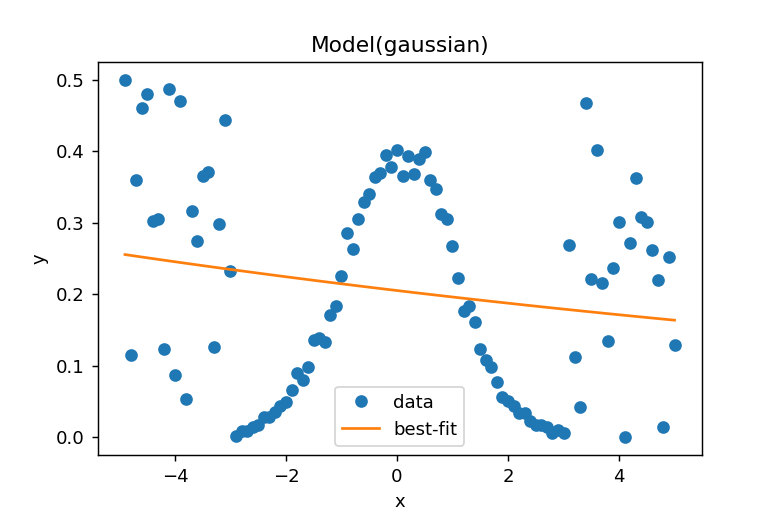
両端のデータに釣られて、うまくフィッティングできていないことがわかる。そこで重み付きによるフィッティングを行う。
重み配列の作成
重みの配列を作成する。両端のデータがおかしいところは重みを小さくする。重み配列はデータと同じ形状でなければならない。
重みを利用したカーブフィット
model.fit()でweightsを指定することで重み付き最小2乗法近似ができる。
重みつきカーブフィットの結果の表示
result.plot_fit()で結果を表示すると以下のようになる。
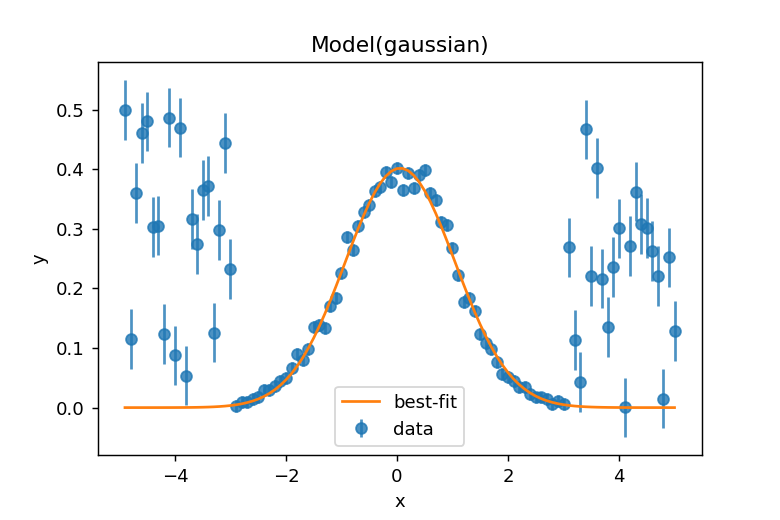
コメント