はじめに
matplotlibのplt.fill_betweenで特定の領域を塗りつぶす際に一部塗りつぶされない領域ができることがある。そこで、interpolate=Trueとしてその領域を塗り潰す方法について説明する。
コード
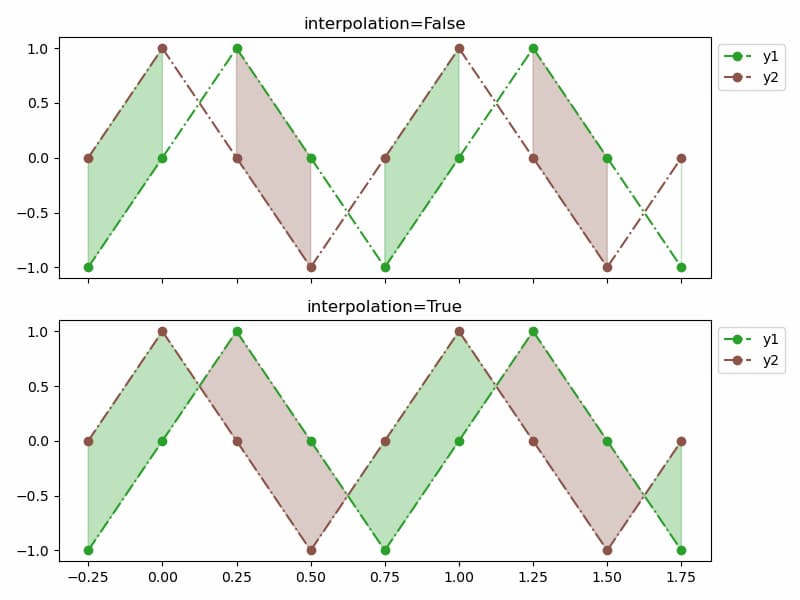
解説
モジュールのインポート
バージョン
データの生成
xを-0.25から1.75の0.25刻みの配列として、np.sin(2 * np.pi * x)でsin波をy1データとする。y1をπ/2だけずらしたデータをy2とする。
上の図(interpolate=Falseの場合)
fill_betweenでwhere=(y1 < y2), color=’C2’とすることでy1<y2の部分を色”C2″で塗りつぶす。y1 < y2の判定はデータポイントで行われるので、データのない部分ではy1<y2であっても塗りつぶされない。
下の図(interpolate=Trueの場合)
fill_betweenでinterpolate=Trueとすることで、where条件が変化するxの値を近似し、その部分まで塗り潰す領域を拡張する。
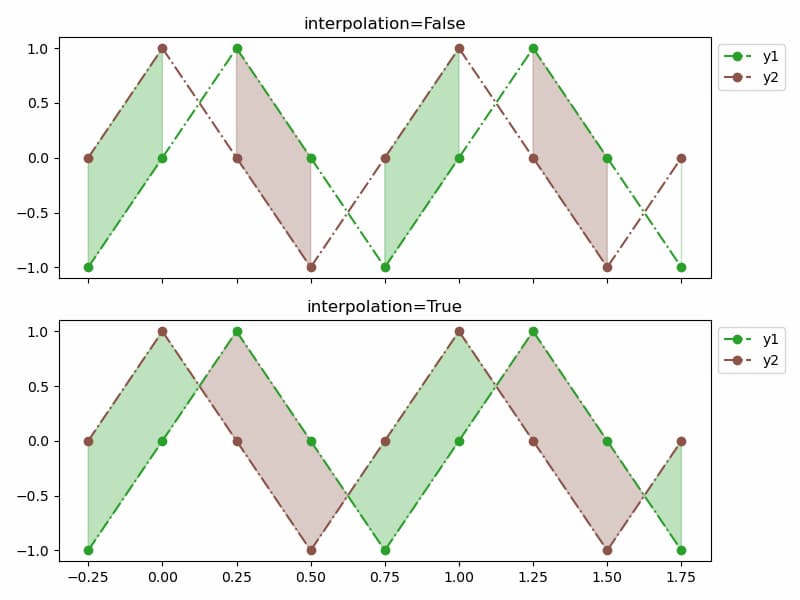
参考
Filling the area between lines — Matplotlib 3.2.1 documentation
matplotlib.axes.Axes.fill_between — Matplotlib 3.2.1 documentation
コメント