はじめに
階層型構造のインデックスのDataFrameにおけるスライスについての解説する。
解説
モジュールのインポート
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import pandas as pd
import numpy as np
pd.options.display.notebook_repr_html = False
マルチインデックスをもつDataFrameの作成
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
data = np.random.randint(0,100,(6, 4))
index = [['a', 'a', 'b', 'b', 'c', 'c'], [1, 2, 1, 2, 1, 2]]
columns = [['data1','data1','data2','data2'],["A","B","A","B"]]
df = pd.DataFrame(data,
index=index,
columns=columns)
df
'''
data1 data2
A B A B
a 1 42 54 28 62
2 26 58 55 0
b 1 81 97 1 59
2 74 95 98 40
c 1 24 46 75 27
2 96 30 45 72
'''
マルチインデックスをもつDataFrameの作成については下記で説明した。
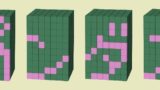
[pandas] 10. マルチインデックスの作成について
Series, DataFrameで使うことのできる階層型構造をもつマルチインデックスの作成についての説明する。
カラム名によるスライス
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
df['data2','A']
'''
a 1 28
2 55
b 1 1
2 98
c 1 75
2 45
Name: (data2, A), dtype: int64
'''
df[‘data2′,’A’]のように最初に上の階層のカラム名、その次に下のカラム名をいれることでデータを取り出すことができる。
.ilocによるスライス
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
df.iloc[:,::2]
'''
data1 data2
A A
a 1 42 28
2 26 55
b 1 81 1
2 74 98
c 1 24 75
2 96 45
'''
df.iloc[:,::2]のように.ilocでpythonの標準的なスライス法を用いることができる。
タプルによるスライス
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
df.loc[['a'],('data2','A')]
'''
a 1 28
2 55
Name: (data2, A), dtype: int64
'''
.locによるスライスでは、タプルを使うこともできる。
これにより、インデックスが[‘a’]で、カラム名が’data2’で’A’であるデータを取り出すことができる。
IndexSliceオブジェクトの利用
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#IndexSliceオブジェクトの利用
idx=pd.IndexSlice
df.loc[idx[:,2],idx[:,'A']]
'''
data1 data2
A A
a 2 26 55
b 2 74 98
c 2 96 45
'''
IndexSliceオブジェクトを利用するとインデックスを単純にスライスした方式で要素へアクセスできる。
コメント