はじめに
scipyのVoronoi,voronoi_plot_2dを使って、ボロノイ領域を塗りつぶしたボロノイ図を表示する方法について説明する。
コード&解説
モジュールのインポート
バージョン
デフォルトのカラーサイクルの変更
デフォルトの”tab10″から”Set3″にカラーサイクルを変更する。
データの作成
半径の異なる円状のデータを作成する。図で表示すると以下のようになる。
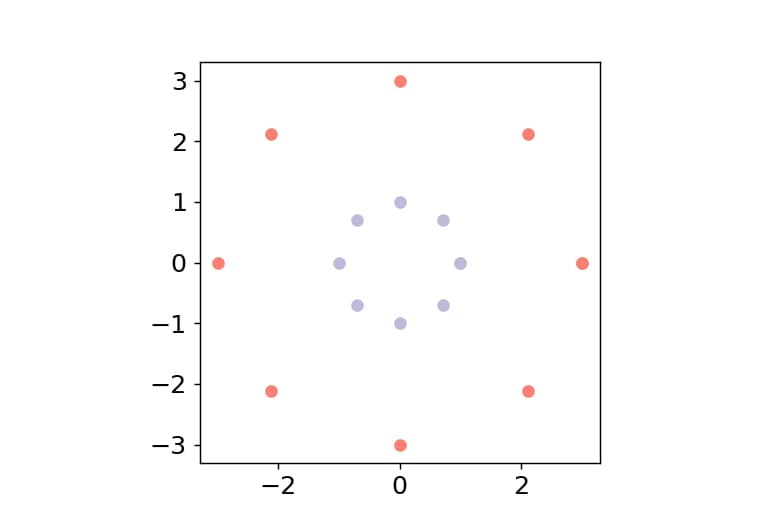
ボロノイ図の作成
配列を結合して、Voronoi
でボロノイ図を表示するためのデータを作成する。
ボロノイ図の表示
ボロノイ図はvoronoi_plot_2d
で表示できる。voronoi_plot_2dについては、下記記事で解説した。
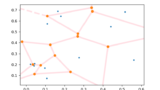
[SciPy] 10. voronoi_plot_2dによるボロノイ図の作成
ボロノイ図とは、任意の位置に配置された複数個の点に対して、同一平面上の他の点がどの点に近いかによって分割した図のことである。ここでは、scipy.spatialのVoronoi, voronoi_plot_2dでボロノイ図を作成する方法を紹介する。
ボロノイ図は下図のようになる。
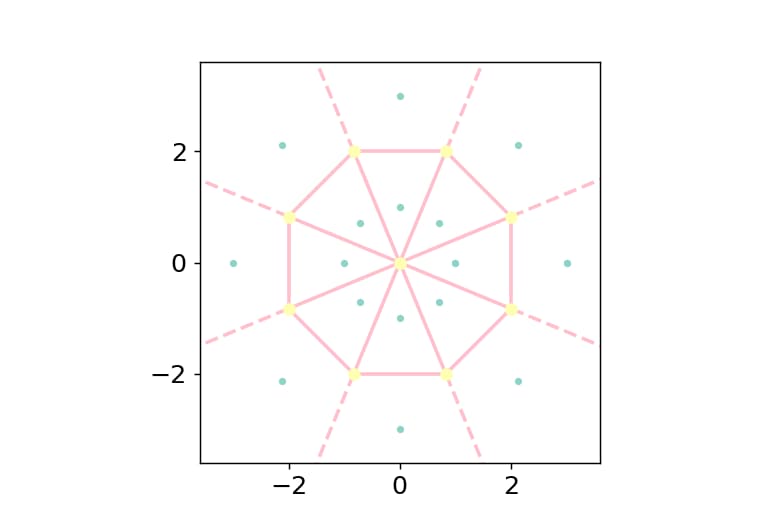
塗りつぶしボロノイ図の表示
vor.regionsで各ボロノイ領域を形成するボロノイ頂点のインデックスを取得し、vor.vertices[region]で塗りつぶす領域を決めて、塗りつぶす。vor.regionsに-1がある場合は、ボロノイ図の外側に頂点があることを意味するので、if -1 not in region:
で塗りつぶさないようにする。
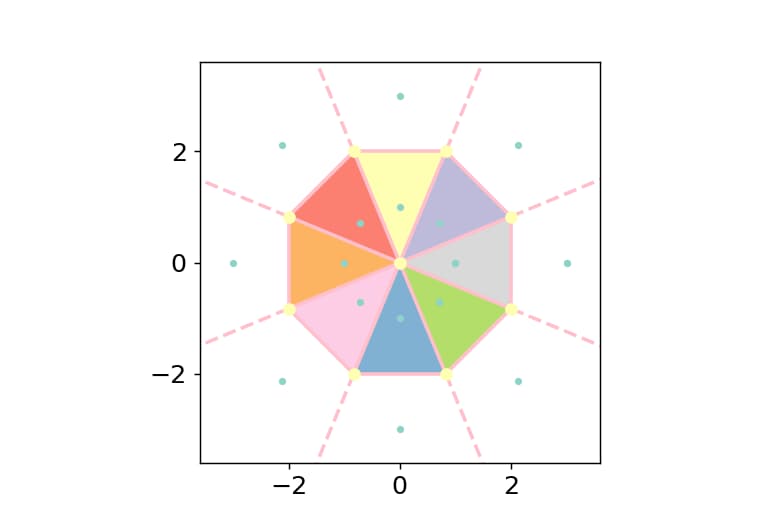
ランダムデータの場合
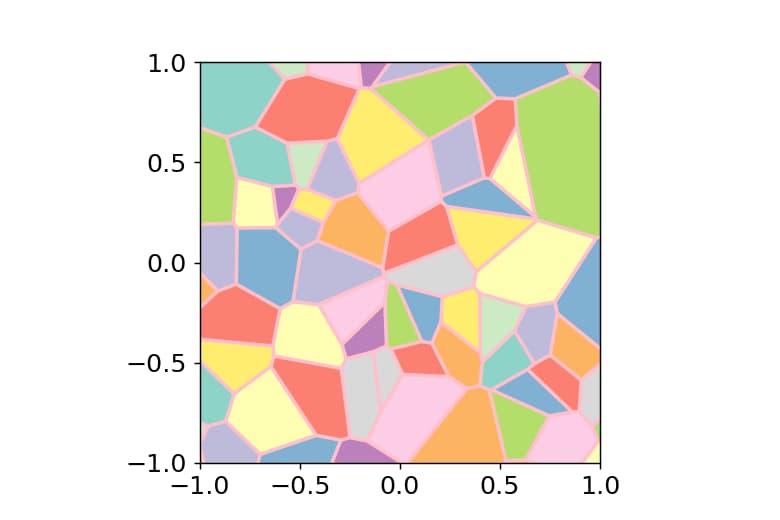
参考

ボロノイ図 - Wikipedia
Voronoi — SciPy v1.15.1 Manual
voronoi_plot_2d — SciPy v1.15.2 Manual
コメント