はじめに
sns.stripplotではカテゴリ形式のデータの散布図をプロットすることができる。その際、プロットの重なりを抑えるために、プロットをランダムに横方向にずらしたプロットをジッタープロットという。ここでは、ジッタープロットのマーカーに画像を適用する例について紹介する。
コード&解説
モジュールのインポートなど
バージョン
データの作成
ランダムデータのDataFrameを作成する。表示すると以下のようになる。
sns.stripplotでジッタープロットを表示
stripplotで jitter=.1とすることでジッタープロットとなる。
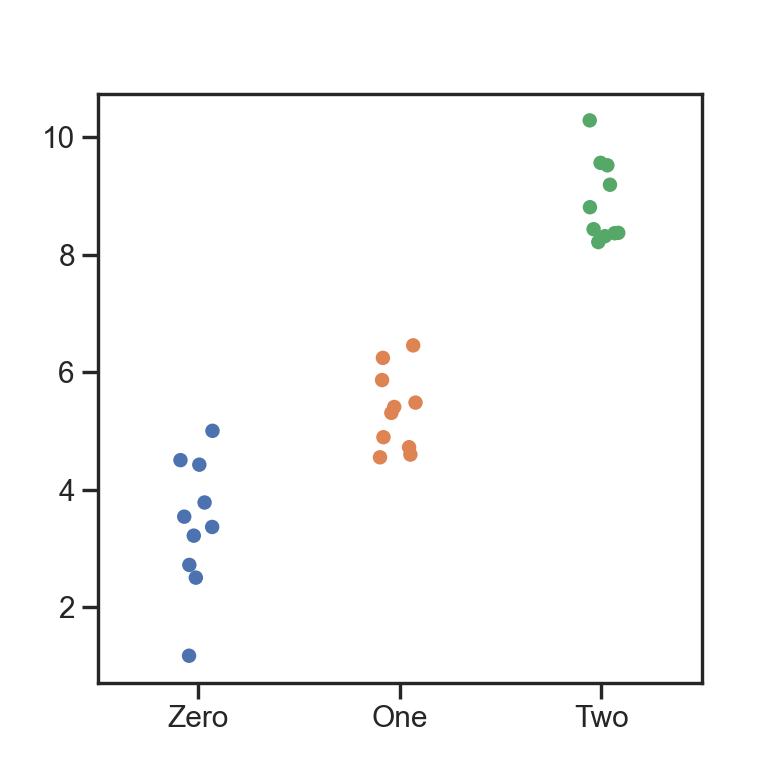
stripplotからデータ取得
p.collections[0].get_offsets().dataのようにすることでstripplotで表示したデータを取得できる。
取得したデータは以下のような形式となっている。
matplotlibのax.plot()で取得したデータを表示
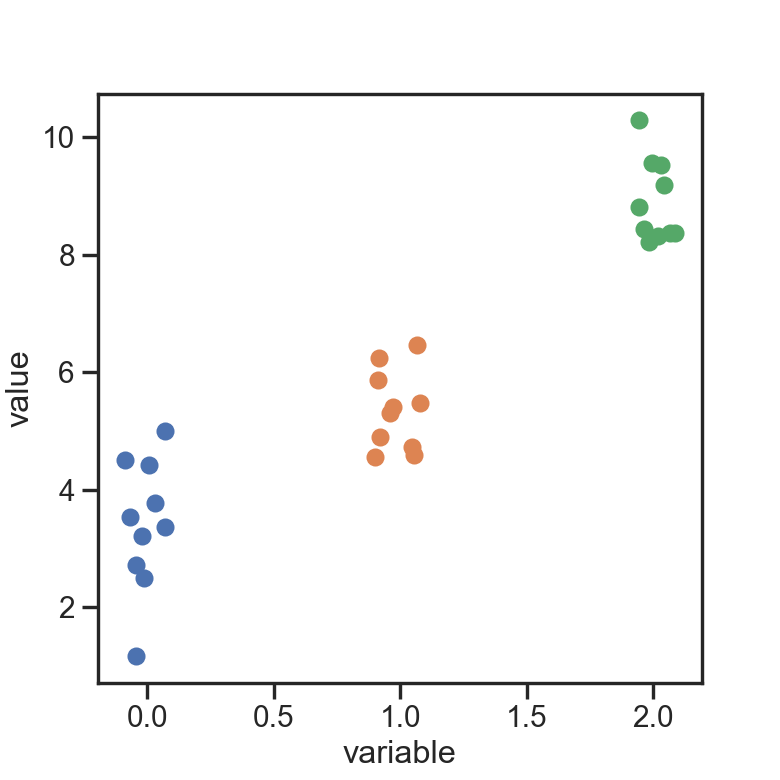
ジッタープロットのマーカーを画像にして表示
画像をプロットする関数
下記ページと同様の関数を用いる。
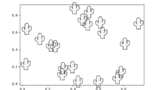
[matplotlib] 3.マーカーを画像にしてプロット
画像ファイルを読み込んでマーカーのかわりにプロットする方法について説明する。
画像ジッタープロットの表示
画像は下記サイトから取得した。

いろいろなジャムのイラスト
いらすとやは季節のイベント・動物・子供などのかわいいイラストが沢山見つかるフリー素材サイトです。
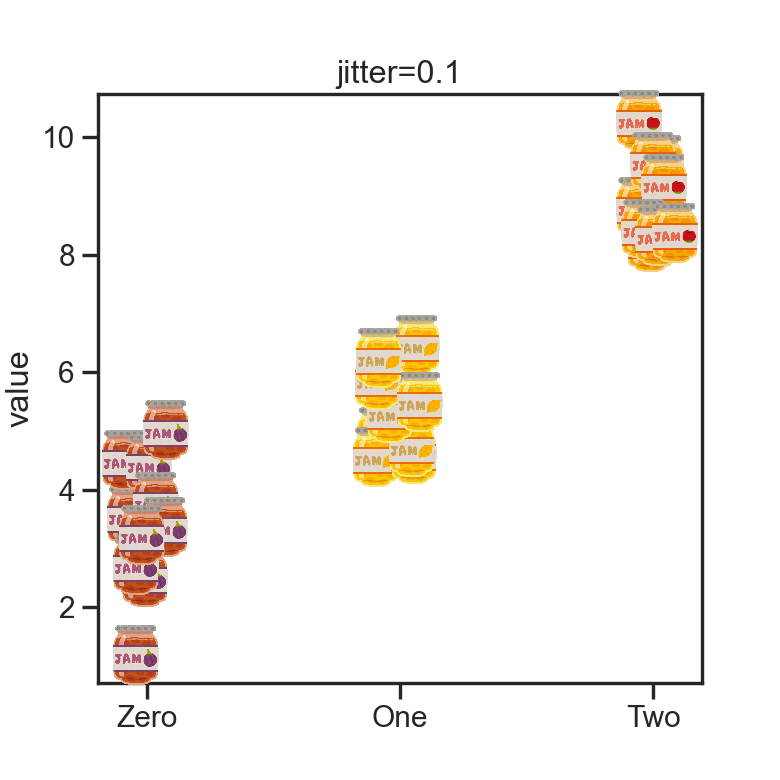
jitter=0.3とした場合
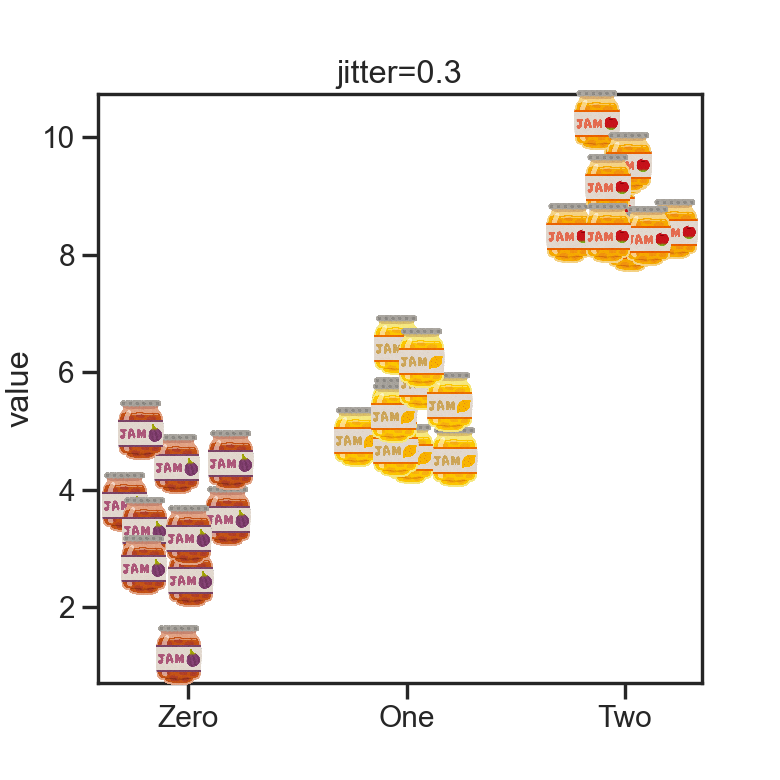
参考
seaborn.stripplot — seaborn 0.13.2 documentation
コメント