はじめに
skimage.transform のpyramid_gaussian, rescaleを用いて、画像の一部分を低解像度(ぼかし加工)化する方法について説明する。
コード
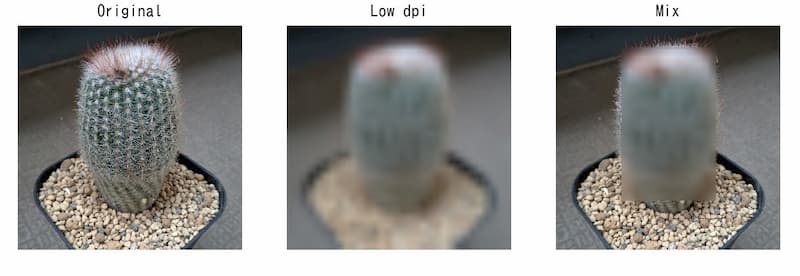
解説
モジュールのインポート
画像の読み込み
パロディア属の紅小町を用いる。画像のサイズは1024 x 1024となっている。
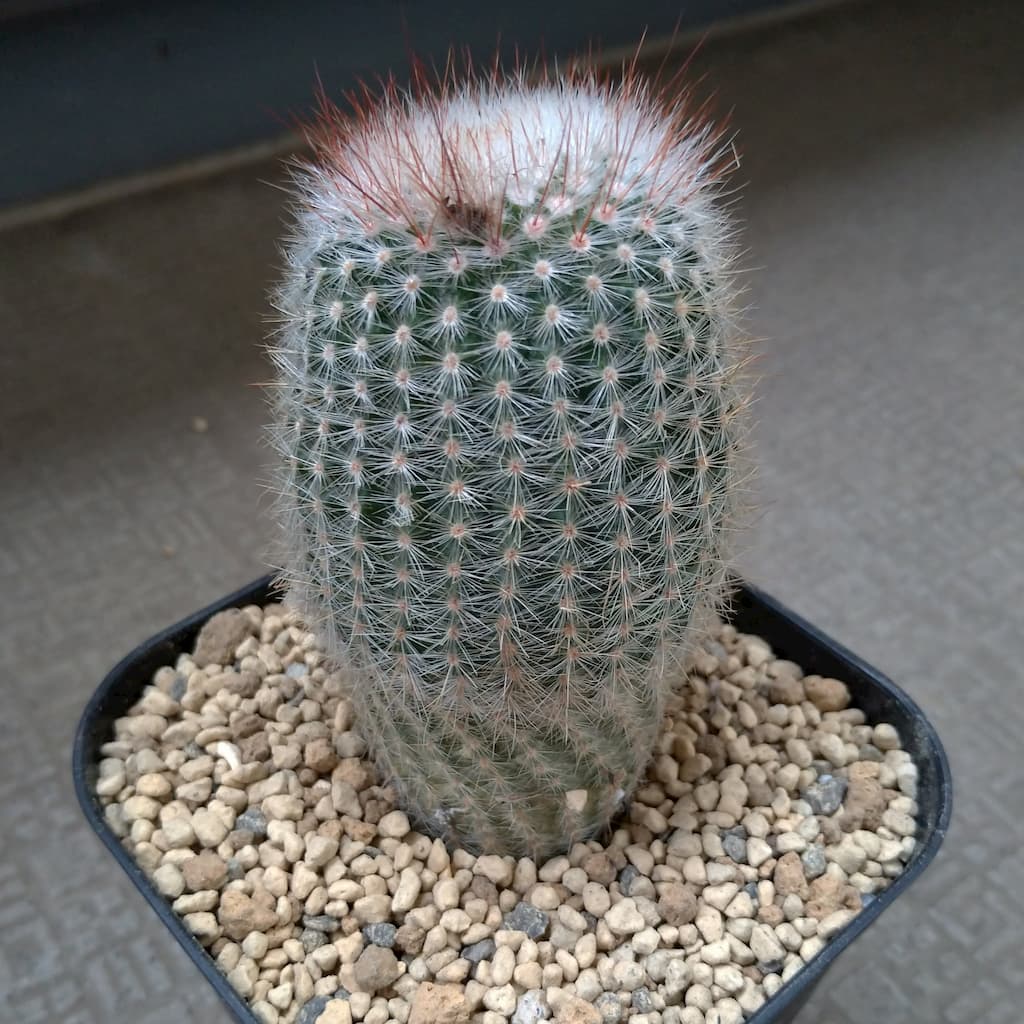
低解像度画像の生成
pyramid_gaussianにより、低解像度の画像を生成する。pyramid_gaussianについては下記で解説した。
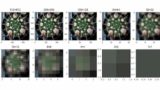
[scikit-image] 25. 一定倍率で縮小された連続画像を生成(transform.pyramid_gaussian)
ここでは、skimage.transformのpyramid_gaussianによる一定倍率で縮小された連続画像の生成方法について説明する。
低解像度化した画像を表示すると下記のようになる。
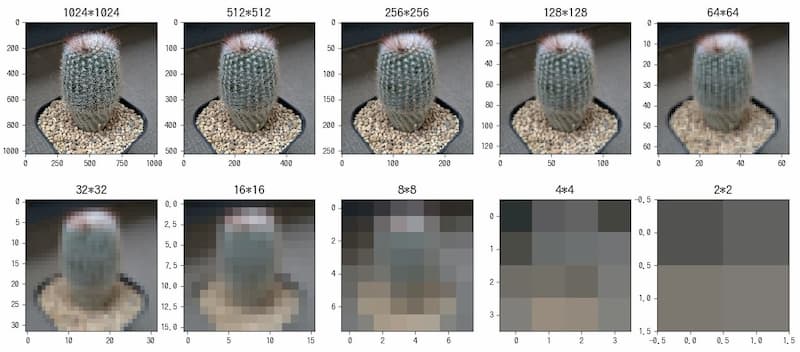
低解像度画像のサイズ変更
rescaleで画像を元画像の大きさまで大きくする。ここではpyramid[5](上図左下の32 x 32の画像)を 2の5乗(32)倍に拡大する。order=1とすることで各画素がスプライン補完され、ぼかしがはいったような画像が得られる。
rescaleした画像はfloat形式(0〜1)となるので、img_as_ubyteで0〜255の値に戻す。
画像の一部の低解像度化
im5[100:800,300:730,:]の部分を低解像度な画像であるimage_rescaled_5[100:800,300:730,:]に置き換える。
結果の表示
左から順に元画像、低解像度画像、サボテンの部分のみを低解像度にした画像となる。
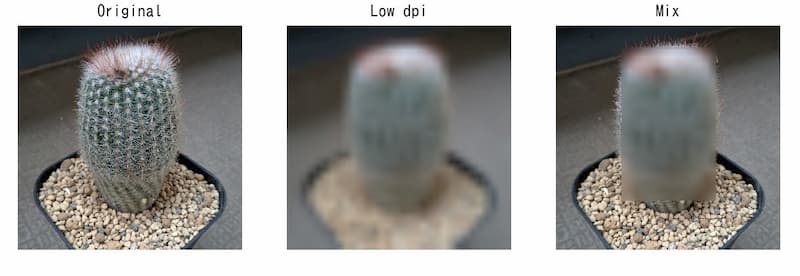
低解像度化のレベルを変えて表示
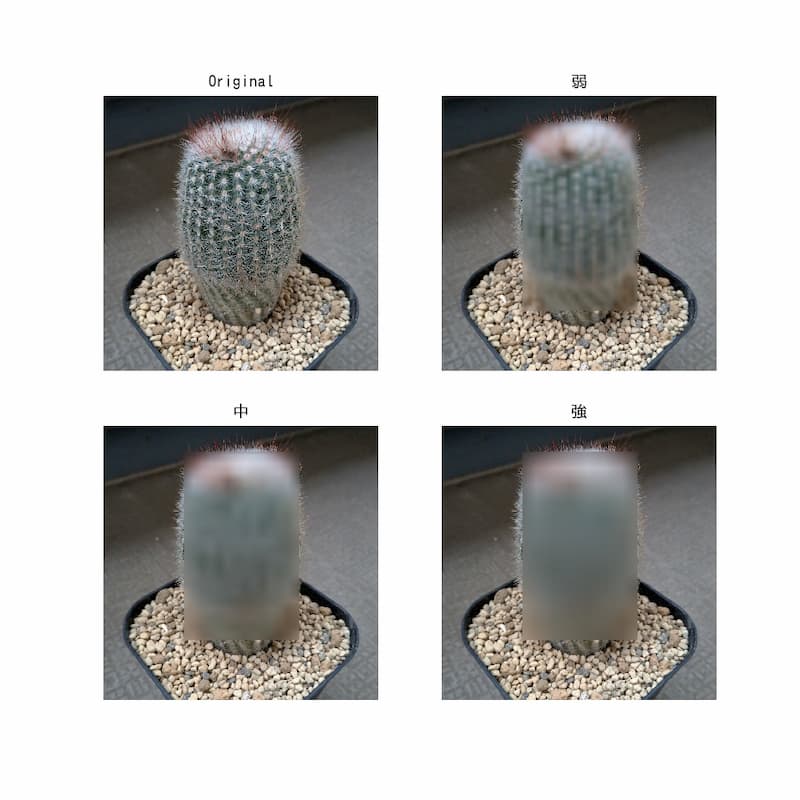
参考
403 Forbidden
skimage.transform — skimage 0.23.3rc0.dev0 documentation
skimage.transform — skimage 0.23.3rc0.dev0 documentation
skimage.util — skimage 0.23.3rc0.dev0 documentation
コメント