はじめに
jupyter notebookの対話的にパラメータを調整できる機能(ipywidgets IntRangeSlider)で、画像の任意の位置に四角をインタラクティブに表示する方法について説明する。
コード
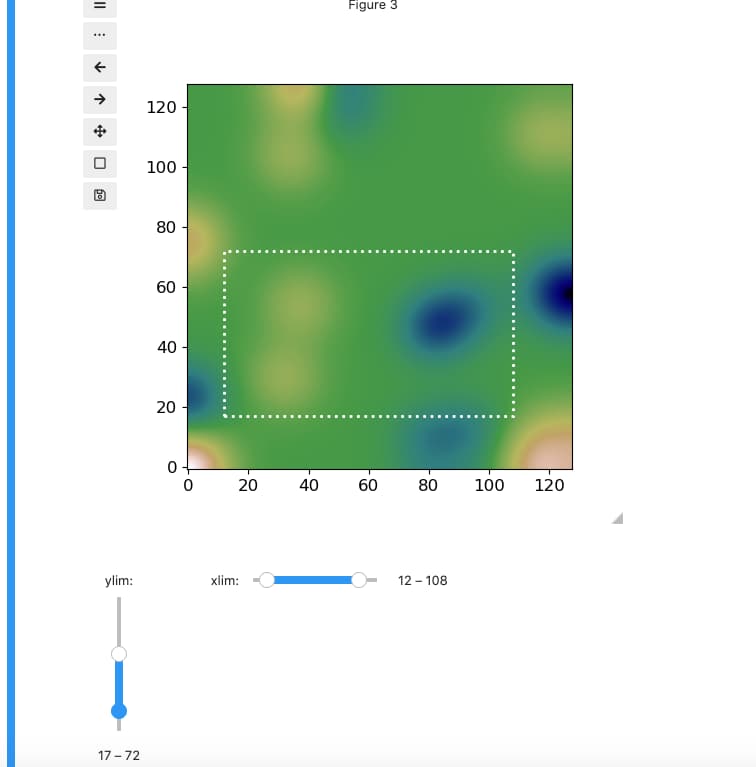
解説
モジュールのインポートなど
バージョン
データの生成
画像データは下記記事と同じコードにより生成した。
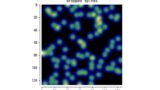
[matplotlib] 57. plt.imshow()で軸を画像から離して表示
matplotlibのplt.imshowで画像を表示する際に、軸を画像から離して表示する方法について説明する。
画像の表示
四角の表示
左下の座標が(0, 0)で幅0、高さ0のRectangleを作成し、ax.add_patch(rects)で図中に入れておく。
ipywidgetsの設定
IntRangeSliderの設定
IntRangeSliderで整数で範囲を指定できる。minは最小値で, maxは最大値、stepは刻み幅でvalueは初期値となる。 descriptionでスライダーの前にラベルをつけることができ、orientation=’horizontal’で水平方向、’vertical’で垂直方向にスライダーを設置するこことができる。
x_lim、y_limのスライダーで選択した範囲に四角が表示されるように、四角の左下位置をset_xyで設定し、幅をset_width、高さをset_heightで設定する。
IntRangeSliderの表示
iinteractではなく、interactiveを用いる。
intaractiveとすることでHBox(children=[y_range,x_range])などのようにipywidgrtsのレイアウト変更が可能となる。
Hboxで横方向にwidgetsが並ぶことになる。
x_lim、y_limを変化させたときの画像の変化
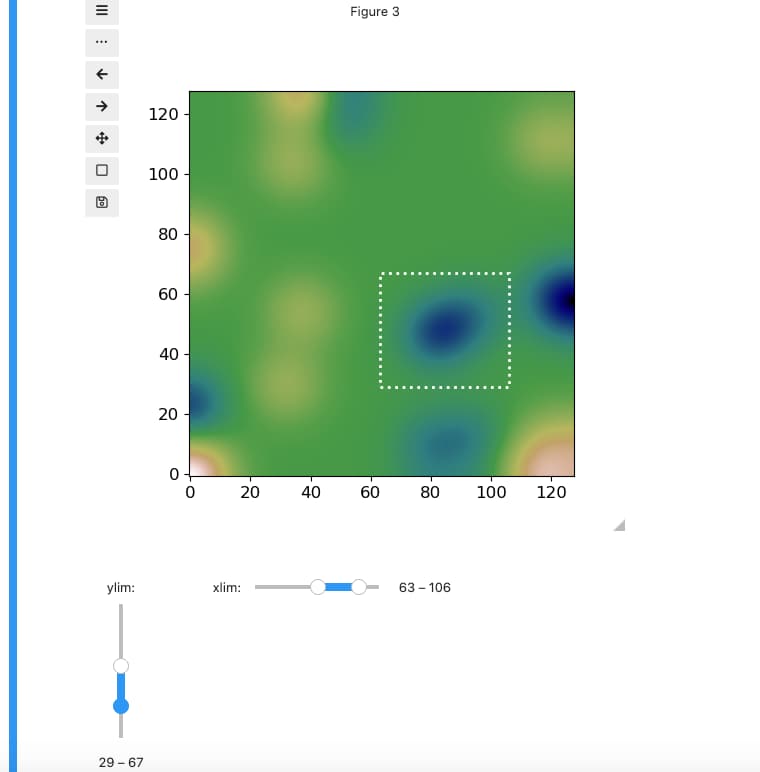
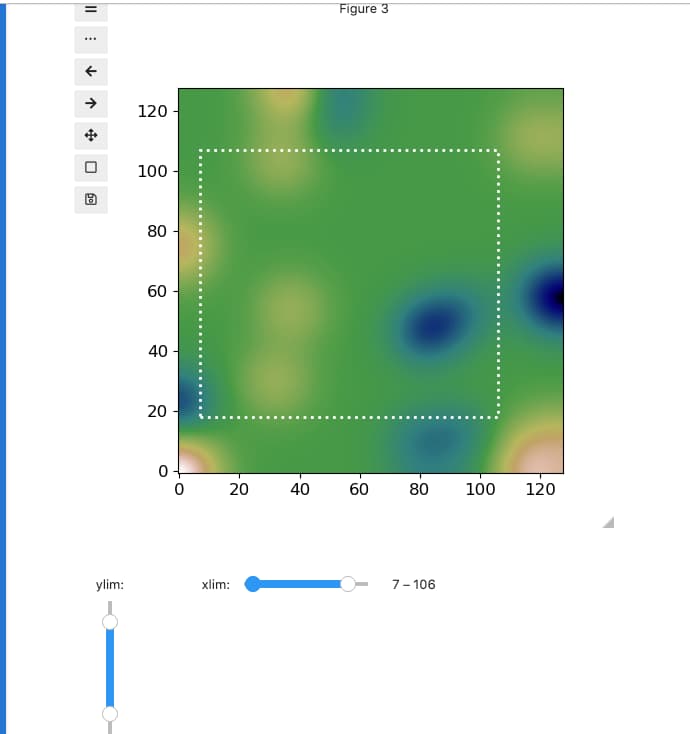
参考
Using Interact — Jupyter Widgets 8.1.7 documentation
Widget List — Jupyter Widgets 8.1.7 documentation
コメント