はじめに
jupyter notebookの対話的にパラメータを選択できる機能(ipywidgets IntSlider)で、scikit-image measureのprofile_lineを使って、画像の任意の位置の強度プロファイルを取得して表示する方法について説明する。
なお、profile_lineについては下記記事で解説した。
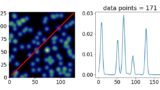
[scikit-image] 71. 画像の強度プロファイルを任意の範囲で表示(skimage.measure profile_line)
skimage.measureのprofile_lineで、任意の範囲の画像の強度プロファイルを表示する方法について説明する。
コード
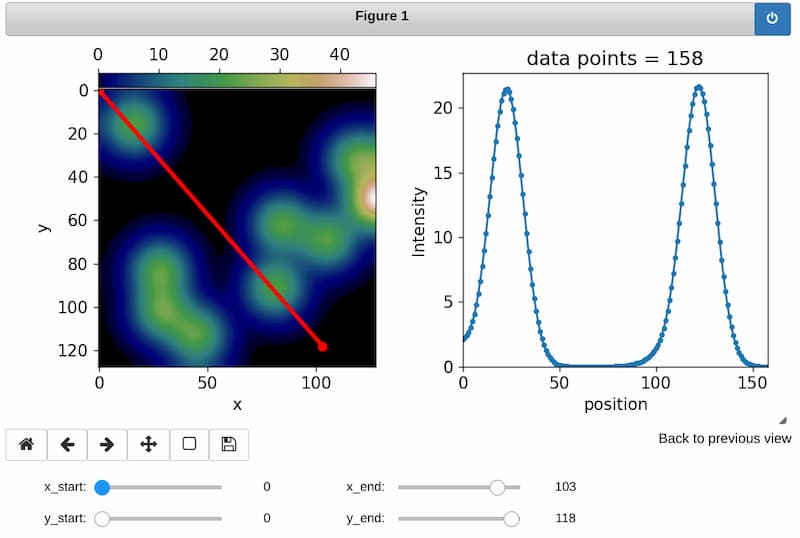
解説
モジュールのインポートなど
画像データの作成
用いる画像については、下記記事と同様に作成した。
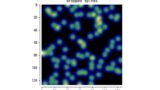
[matplotlib] 57. plt.imshow()で軸を画像から離して表示
matplotlibのplt.imshowで画像を表示する際に、軸を画像から離して表示する方法について説明する。
画像の表示
make_axes_locatableでカラーバーを画像の上に表示した。cax.xaxis.set_ticks_position(‘top’)でカラーバーの目盛りが上側に来るようにした。
ipywidgetsの設定
plotの作成
widgetsで取得した値を用いてプロットを表示するためにデータが空のプロットを作成しておく。
proはskimageのprofile_lineで取得したデータを表示するためにプロットであり、画像の横のax[1]に表示する。
IntSliderの作成
プロファイルの開始点の座標と終了点の座標を取得するためにIntSliderを作成する。
Sliderの値を変えたときに行う処理
set_dataで選択した値を用いて、画像上にプロファイルを取得する範囲を点と線で示す。
profile_lineに取得した座標を入れて、プロファイルを得る。得たプロファイルも同様にset_dataでデータをセットすることで表示する。
Widgetsの有効化と表示
interactiveで関数fを有効にし、ipywidgetsが動作するようにする。
widgetsはHBox とVBoxを用いて、整列させたものを作成して、それをdisplay(ui)で表示した。
IntSliderを変化させたときの強度プロファイルの変化
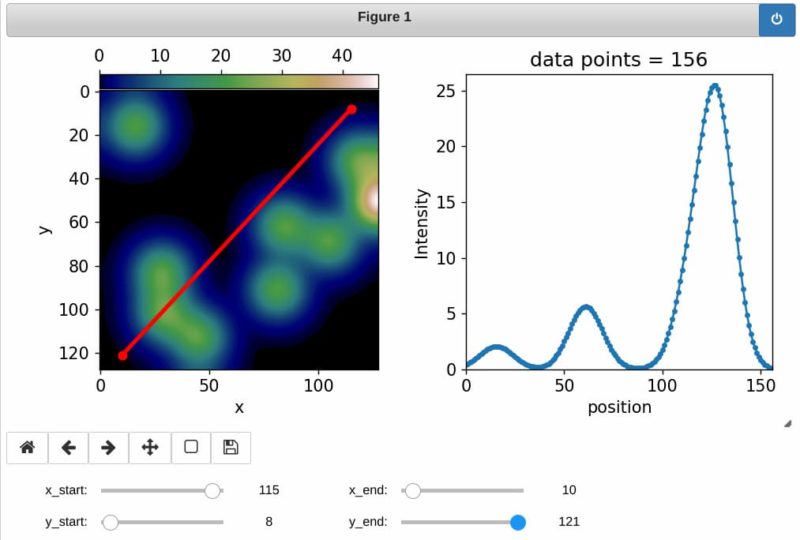
参考
Using Interact — Jupyter Widgets 8.1.7 documentation
Widget List — Jupyter Widgets 8.1.7 documentation
コメント