matplotlib FuncAnimationによるVoxelグラフのアニメーション
移動アニメーション
コード
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
import matplotlib.animation as animation
from IPython.display import HTML
import numpy as np
#move anim
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.set_aspect('equal')
def update(num):
ax.cla()
ax.set_xlabel("x")
ax.set_ylabel("y")
ax.set_zlabel("z")
voxels = (x < num+1) & (y < num+1) & (z < num+1) & (x > num-1) & (y > num-1) & (z > num-1)
ax.voxels(voxels, facecolors='g', edgecolor='k')
x, y, z = np.indices((10, 10, 10))
ani = animation.FuncAnimation(fig, update, 10, interval=100)
HTML(ani.to_html5_video())
解説
モジュールのインポート
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
import matplotlib.animation as animation
from IPython.display import HTML
import numpy as np
3Dグラフの設定
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.set_aspect('equal')
座標データの生成
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
x, y, z = np.indices((10, 10, 10))
np.indices()で形状が(10,10,10)の配列で要素としてインデックスをもつ配列を作成。
アニメーションの設定
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
def update(num):
ax.cla()
ax.set_xlabel("x")
ax.set_ylabel("y")
ax.set_zlabel("z")
voxels = (x < num+1) & (y < num+1) & (z < num+1) & (x > num-1) & (y > num-1) & (z > num-1)
ax.voxels(voxels, facecolors='g', edgecolor='k')
ax.cla()で古いボクセルグラフを消去する。
その際、ラベルも消えてしまうので、毎回ax.set_xlabel()などでラベル名を設定。
ボクセルは配列中のTrueの位置にだけ表示される。
voxels = (x < num+1) & (y < num+1) & (z < num+1) & (x > num-1) & (y > num-1) & (z > num-1)
とすることでTrueの位置が(0,0,0),(1,1,1),(2,2,2)と変化していくので、3Dグラフ上でボクセルが移動するアニメーションが得られる。
アニメーションの表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
ani = animation.FuncAnimation(fig, update, 10, interval=100)
HTML(ani.to_html5_video())
FuncAnimationでanimationを表示する。updateを10step実行してアニメーションとする。intervalは100 ms なので1秒のアニメーションとなる。
HTML(ani.to_html5_video())でjupyter notebook上にアニメーションを表示できる。
膨張アニメーション
コード
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.set_aspect('equal')
def update(num):
ax.cla()
ax.set_xlabel("x")
ax.set_ylabel("y")
ax.set_zlabel("z")
voxels = (x < 11+num) & (y < num+11) & (z < num+11) & (x > 9-num) & (y > 9-num) & (z > 9-num)
ax.voxels(voxels, facecolors='g', edgecolor='k')
x, y, z = np.indices((20, 20, 20))
ani = animation.FuncAnimation(fig, update, 10, interval=200)
HTML(ani.to_html5_video())
解説
アニメーションの設定
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
voxels = (x < 11+num) & (y < num+11) & (z < num+11) & (x > 9-num) & (y > 9-num) & (z > 9-num)
ax.voxels(voxels, facecolors='g', edgecolor='k')
voxels = (x < 11+num) & (y < num+11) & (z < num+11) & (x > 9-num) & (y > 9-num) & (z > 9-num)
のようにすることで、Trueの位置が(10,10,10)から周りに1個ずつボクセルが増えていくアニメーションができる。
積み上げアニメーション
コード
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
fig = plt.figure()
ax = fig.gca(projection='3d')
def update(num):
ax.cla()
ax.set_xlabel("x")
ax.set_ylabel("y")
ax.set_zlabel("z")
v1 = (x == 2) & (y == 2) & (z < num)
v2 = (x == 7) & (y == 7) & (z < num)
v3 = (x == 2) & (y == 7) & (z < num)
v4 = (x == 7) & (y == 2) & (z < num)
voxels = v1 | v2 | v3 | v4
colors = np.empty(voxels.shape, dtype=object)
colors[v1] = 'red'
colors[v2] = 'blue'
colors[v3] = 'gold'
colors[v4] = 'green'
ax.voxels(voxels, facecolors=colors, edgecolor='k')
x, y, z = np.indices((10, 10, 10))
ax.set_aspect('equal')
ani = animation.FuncAnimation(fig, update, 10, interval=200)
HTML(ani.to_html5_video())
解説
アニメーションの設定
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
v1 = (x == 2) & (y == 2) & (z < num)
v2 = (x == 7) & (y == 7) & (z < num)
v3 = (x == 2) & (y == 7) & (z < num)
v4 = (x == 7) & (y == 2) & (z < num)
voxels = v1 | v2 | v3 | v4
colors = np.empty(voxels.shape, dtype=object)
colors[v1] = 'red'
colors[v2] = 'blue'
colors[v3] = 'gold'
colors[v4] = 'green'
ax.voxels(voxels, facecolors=colors, edgecolor='k')
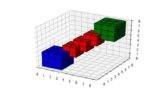
[matplotlib 3D] 28. Pythonで3D voxelグラフ
3D voxelグラフの作成について解説する。
↑を参考にして、各ボクセルの色が異なるアニメーションを作成することもできる。
参考
3D voxel / volumetric plot — Matplotlib 3.10.1 documentation
301 Moved Permanently
コメント