はじめに
matplotlibのFuncAnimationで3次元のランダムウォークアニメーションを表示する。
コード
解説
モジュールのインポート
3Dグラフなので from mpl_toolkits.mplot3d import Axes3D
とする。
バージョン
データの生成
3次元のx,y,zデータを極座標系で媒介変数によって生成する。このデータ生成方法は下記でも用いた。
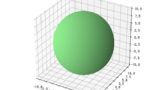
x,y,zをvstackでひとまとめにし、.Tによって転置する。
np.linalg.norm()でaxis=1とすることで各行における要素のノルムを求める。
求めたノルムで各要素をわることで各行の要素を規格化する。
np.vstack(([[0,0,0]],xyz))によりスタート地点を[0,0,0]とした。
positionは下記の1Dランダムウォーク、2Dランダムウォークの例と同様にnp.cumsum()により、累積和とすることで順次変化するランダムウォークの位置を取得した。
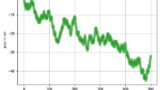
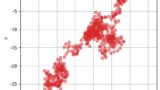
3次元のランダムウォークデータの表示
上記で作成したデータを表示すると以下のようになる。
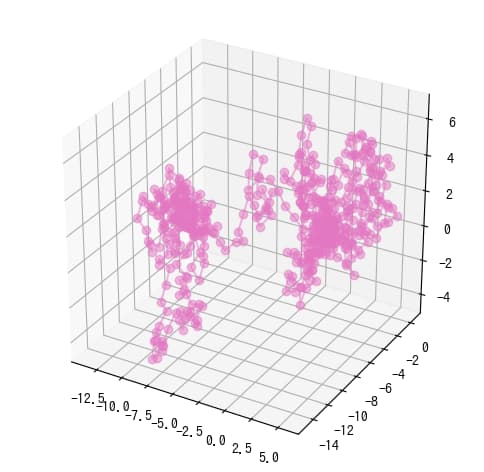
上の図のx軸、y軸、z軸の表示範囲をax.get_xlim(), ax.get_ylim(), ax.get_zlim()で取得する。
アニメーションの表示
p1,で空のプロットを作成して、データを流し込んでいく。
x,yデータのセットには、set_dataを用いる。zデータのセットには、set_3d_propertiesを用いる。
x軸, y軸、z軸の表示範囲は、ax.set(xlim=xlim, ylim=ylim, zlim=zlim)のようにして先程取得したものを用いる。
ani = animation.FuncAnimationとし、このaniをHTML(ani.to_html5_video())とすることでjupyter lab(notebookも)上でアニメーションを表示できる。
コードをダウンロード(.pyファイル) コードをダウンロード(.ipynbファイル)
コメント