はじめに
matplotlibのmplot3dの3Dボクセルグラフで立体的なハートを表示する。
コード
ハート
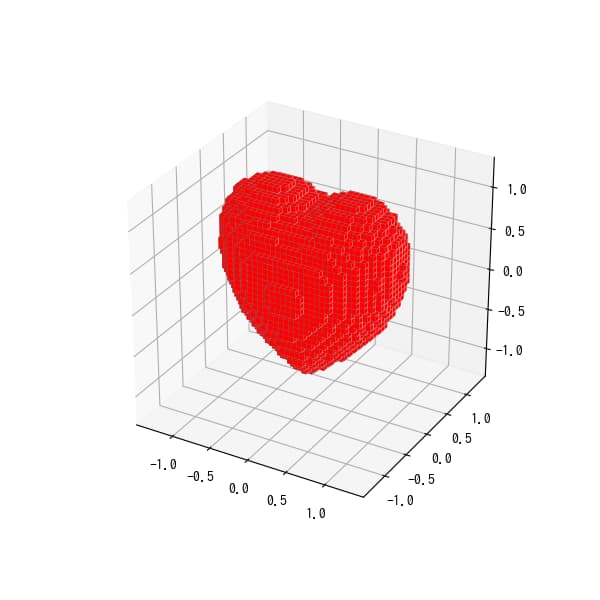
回転アニメーション
解説
モジュールのインポート
データの生成
データの生成については、下記のトーラスと同様にして生成した。
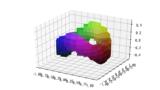
[matplotlib 3D] 31. voxels_torus
matplotlib mplot3dのvoxelでトーラスを表示。
heartの3D形状は以下の式で表される。[1]
$$(x^2 + \frac{4}{9}y^2+z^2-1)^3 -x^2z^3+ -\frac{9}{80}y^2z^3 = 0$$<0とすることでハートの部分だけボクセルを表示することができる。
ハートの表示
アニメーションの表示
アニメーションのコードは下記のwireframeの回転アニメーションとおなじコードとなっている。
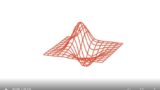
[matplotlib 3D] 14. 3Dグラフの回転アニメーション
matplotlib mplot3d のグラフの回転アニメーション
参考
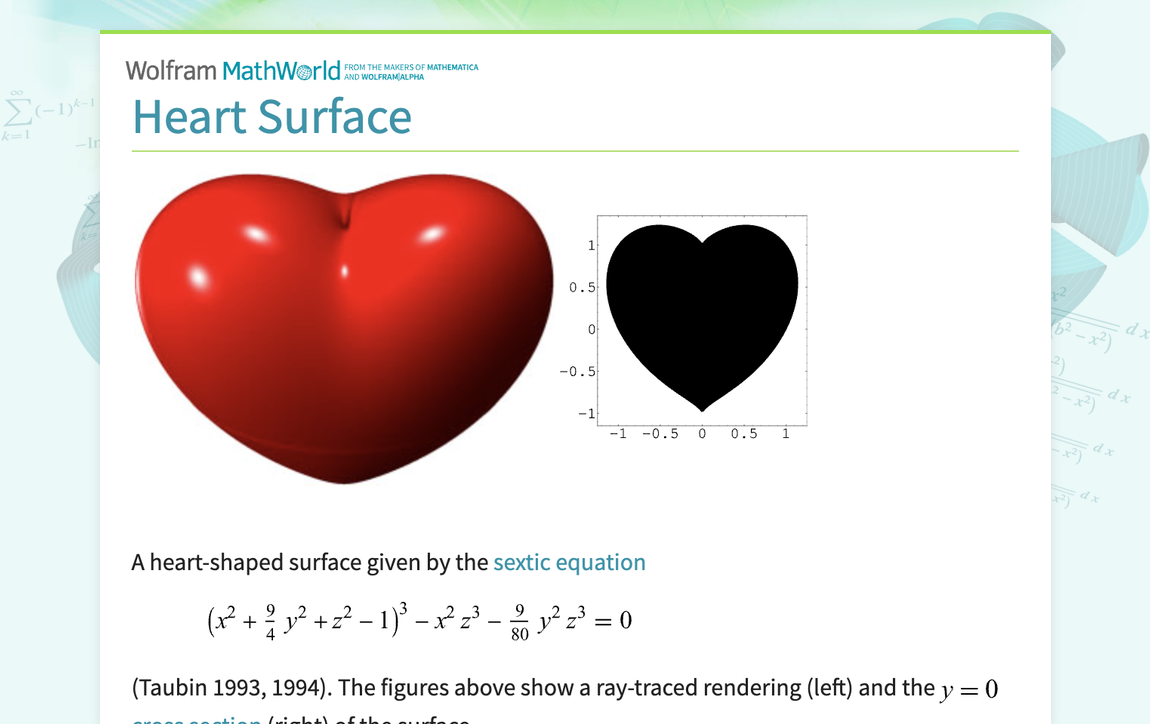
Heart Surface -- from Wolfram MathWorld
A heart-shaped surface given by the sextic equation (x^2+9/4y^2+z^2-1)^3-x^2z^3-9/(80)y^2z^3=0 (Taubin 1993, 1994). The ...
コメント