はじめに
ここでは、skimage.utilのpadによる画像の周囲を補間する方法について説明する。
コード
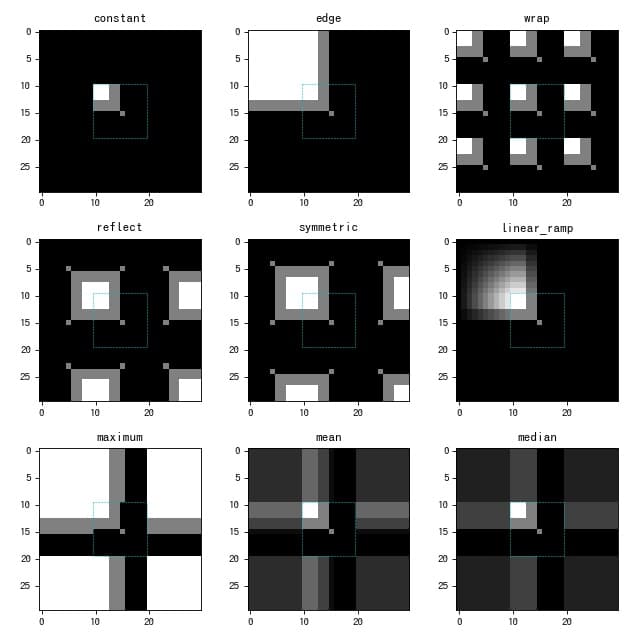
解説
モジュールのインポート
画像データの作成
図の作成
画像の補間
modesのリストに示した方法で補間する。
pad()により、画像の補間ができる。pad_widthをimg[0].shape(10)としているので、補間された画像のサイズは(30,30)となる。
ax[n].plot([9.5, ・・・, 9.5], ‘c–‘, linewidth=0.5)で、元の画像の部分にシアン色の線を表示する。
補間のモード
‘constant’
定数値で補間する。
‘edge’
エッジの値で補間する。
‘wrap’
画像と同様の画像で補間する。
‘reflect’
各軸で反射した画像になるように補間される。
‘symmetric’
各軸に対して対称的になるように補間される。
‘linear_ramp’
end_valueとエッジの値の間での線形補間となる。
‘maximum’
各軸に対して、ベクトルのすべて、または、一部の最大値で補間される。
‘mean’
各軸に対して、ベクトルのすべて、または、一部の平均値で補間される。
‘median’
各軸に対して、ベクトルのすべて、または、一部の中央値で補間される。
図のアスペクト比を揃える設定
参考
Interpolation: Edge Modes — skimage 0.25.0 documentation
コメント