はじめに
skimage.morphologyのstarで、大きさの異なる星形の構造化要素を作成して表示する。
コード
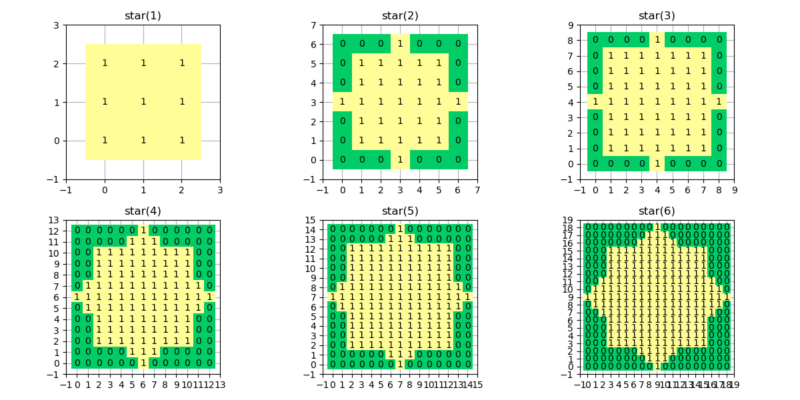
解説
モジュールのインポートなど
バージョン
構造化要素の生成
star(5)でサイズ5の正方形とその正方形が45度回転したものが重なった形状となる。
構造化要素の表示
plt.figure(figsize=(12,6))でfigを作成し、fig.add_subplot(2, 3, idx)で図を順次生成していく。ax.imshow(struc)で構造化要素を表示する。
2重のfor文とax.textにより各ピクセルの中央に各ピクセルの値を表示する。
ax.xaxis.set_major_locator(ticker.MultipleLocator(1))とすることで1ごとに目盛りを表示する。
この状態でax.grid()をすると、1ごとにグリッドが表示されることになる。
ax.set(xlim=(-1,struc.shape[0]),ylim=(-1,struc.shape[0]))で各構造化要素の大きさに応じた軸範囲設定を行う。
ax.set_axisbelow(True)によりグリッドを再下面に表示する。
idx += 1によりidxに1を足して次の図の作成を行う。
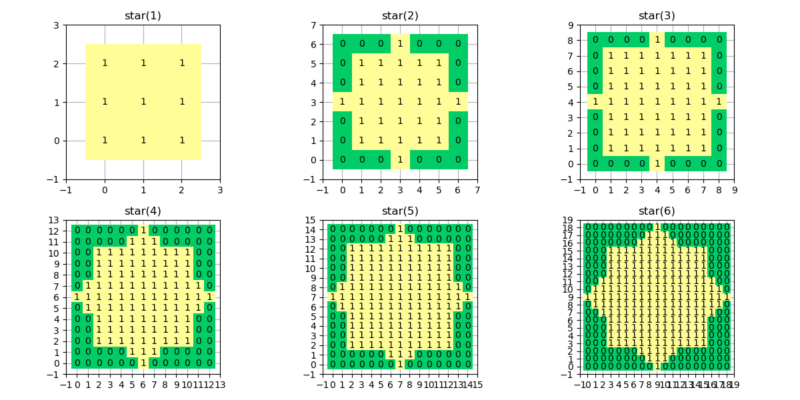
参考
Generate footprints (structuring elements) — skimage 0.25.2rc0.dev0 documentation
skimage.morphology — skimage 0.25.2rc0.dev0 documentation
コメント