はじめに
ヒストグラムを比較する際、複数のヒストグラムを重ねて表示することが多い。しかし、ヒストグラムが重なり合っている場合、分布がどの程度まで及んでいるのかがわからなくなる。したがって、ここでは棒グラフのalpha(透明度)を調整することでヒストグラムの視認性を高める方法について説明する。
コード
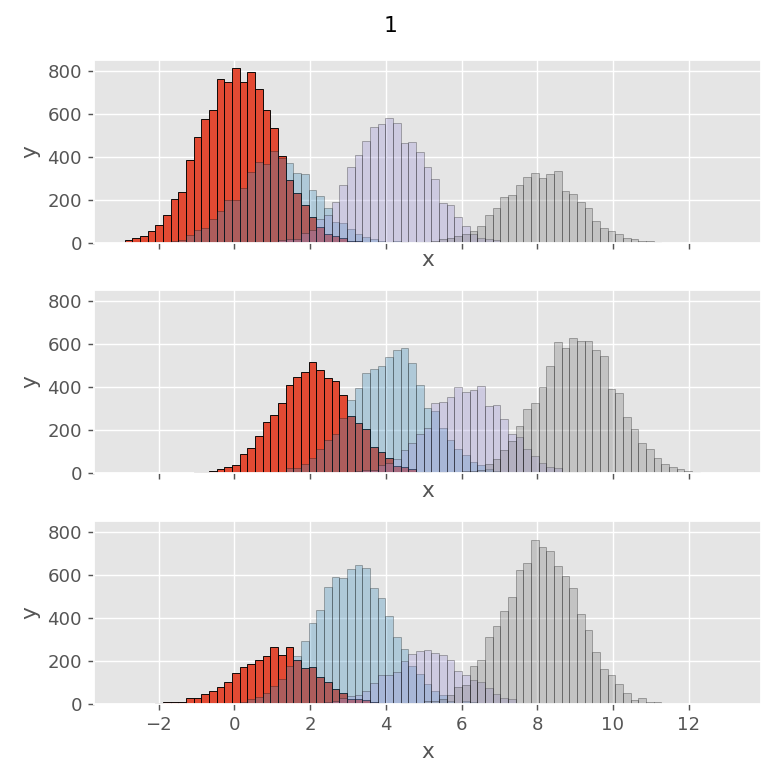
解説
モジュールのインポートなど
バージョン
データの生成
np.random.randn()で0から1の範囲の乱数を作成する。
続いて、np.histogramでヒストグラムデータに変換する。
全て不透明なヒストグラムの場合
全てのヒストグラムのalphaを1とし、不透明とした場合は以下のようになる。
ヒストグラムが互いに重なり合い、見えない部分が発生している。
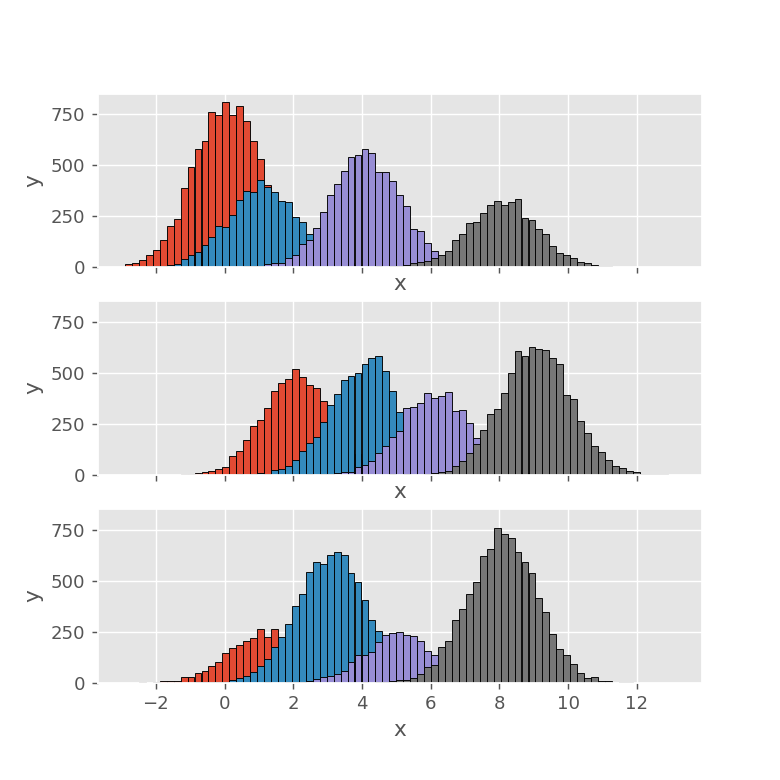
ヒストグラムのalphaを調整した場合
一つのヒストグラムを不透明にし、その他を透明とした場合は以下のようになる。重なりあっている部分があらわになっていることがわかる。
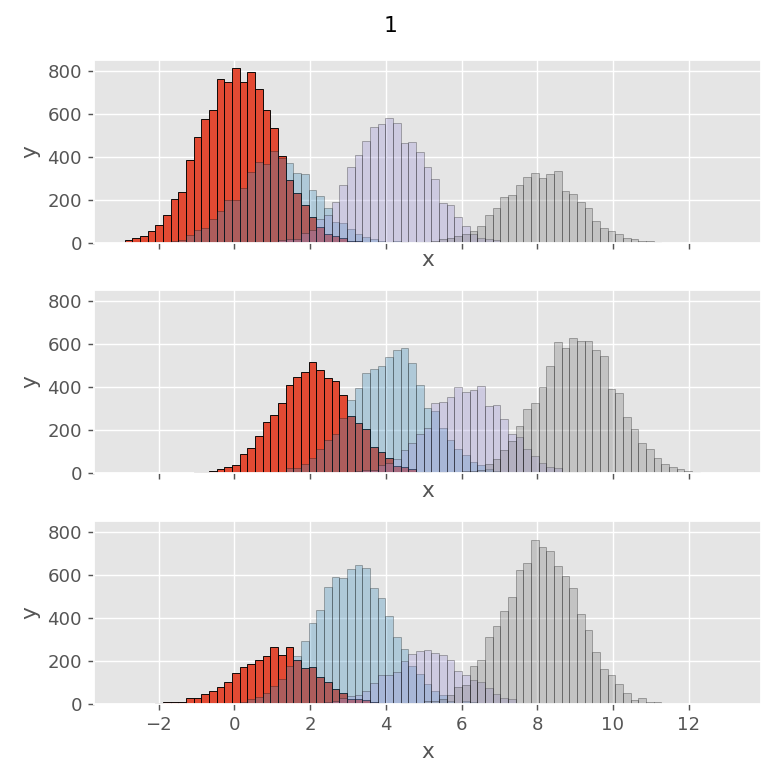
不透明なヒストグラムが最前面に来る場合があるので、その際はzorderを設定して、不透明なヒストグラムが最背面に来るようにする。
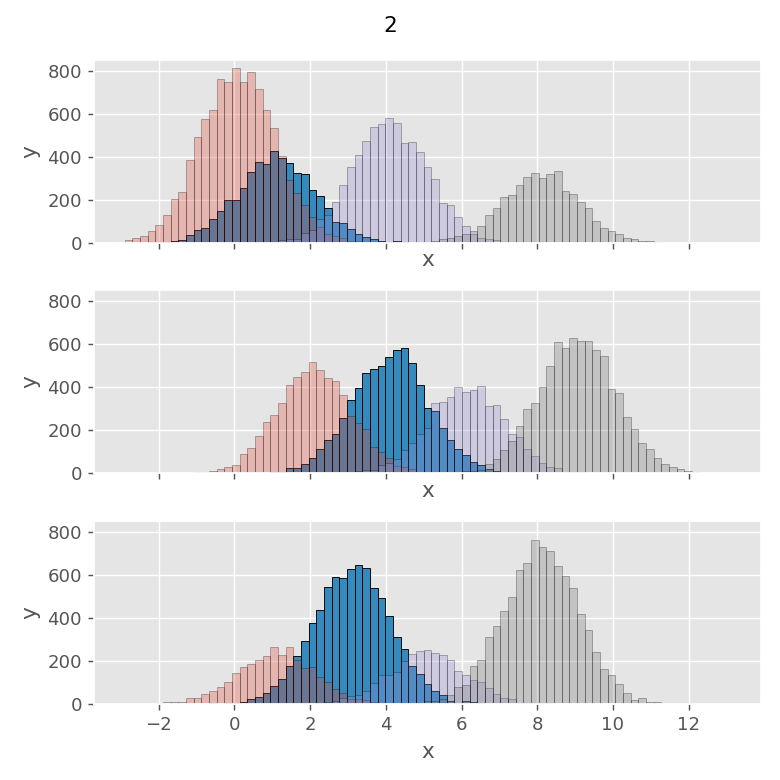
参考
numpy.histogram — NumPy v2.3 Manual
matplotlib.pyplot.bar — Matplotlib 3.10.3 documentation
コメント