はじめに
jupyter notebookでは対話的にパラメータを調整できる機能(ipywidgets)がある。ここでは、その機能の一つであるinteractにより、ヒストグラム上でしきい値を動かし、2値化像を変化させる方法について説明する。
コード
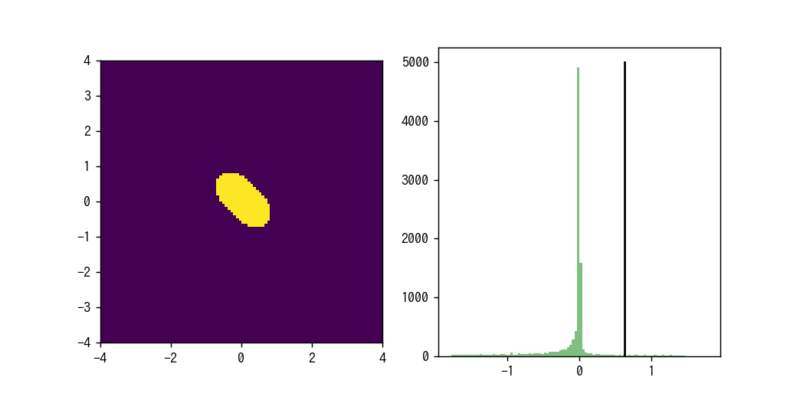
解説
モジュールのインポート
データの生成
2値化に用いる画像
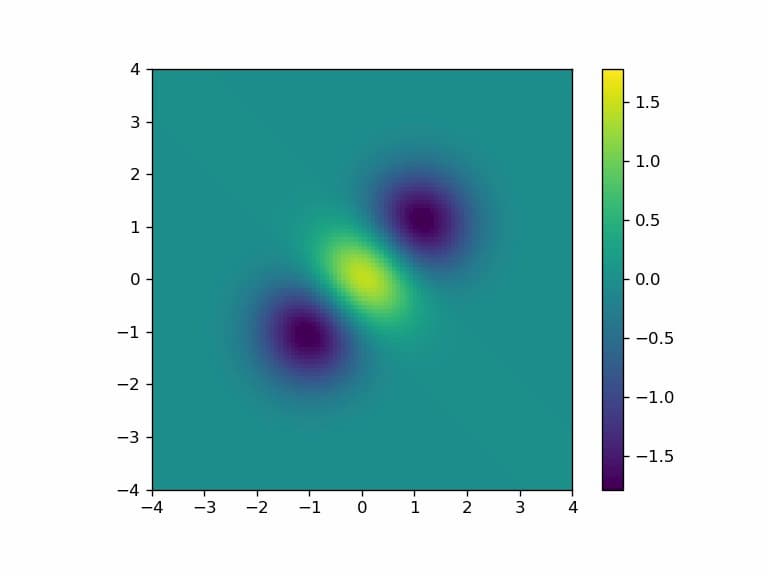
この画像を2値化する。この画像はmatplotlibのSliderで用いた画像と同じとなっている。
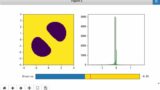
[matplotlib] 38. widgets.Sliderによる画像の2値化
matplotlibではインタラクティブにパラメータを調整できる機能がある。ここでは、その機能の一つであるwidgets.Sliderにより、ヒストグラム上でしきい値を動かし、2値化像を変化させる方法について説明する。
ヒストグラムの設定
plt.subplots(1, 2)とすることで横に並んだ図を作成し、左の図であるax1に2値化像、右の図のax2にヒストグラムを表示する。ヒストグラムはax.hist()で作成した。
l, = ax2.plot((ini,ini),(0,5000),’k-‘)によって、ヒストグラム上にしきい値の値を示す垂線を表示する。
interactの設定
2値化はbinarized = Z>xで行う。これでxより大きい要素がTrueとなってそれ以外の要素はFalseとなる。
ax1.imshow(binarized, interpolation=’None’,origin=’lower’, extent=[-4, 4, -4, 4])により2値化像を表示する。
l.set_xdata((x,x)) により、しきい値を示す垂線を移動する。
interactの適用
interact(f, x=(vmin,vmax))により、関数f(x) のxの値をvminからvmaxの間で変化させることができるスライダーが出現して、スライダーで値を設定するごとに関数f(x)が実行される。
スライダーを動かしたときの変化
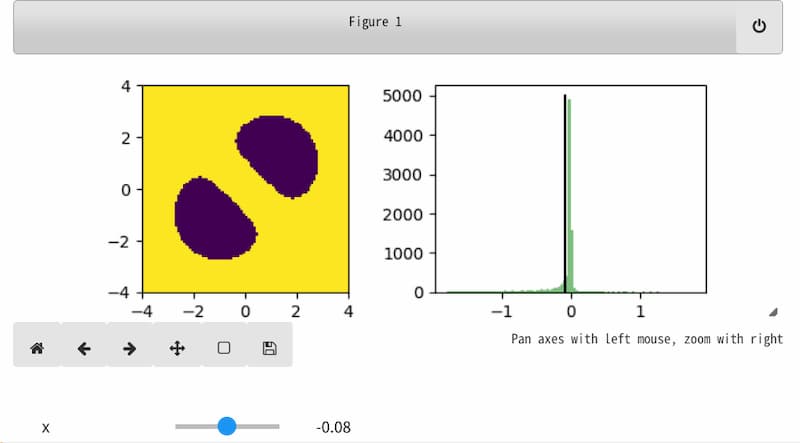
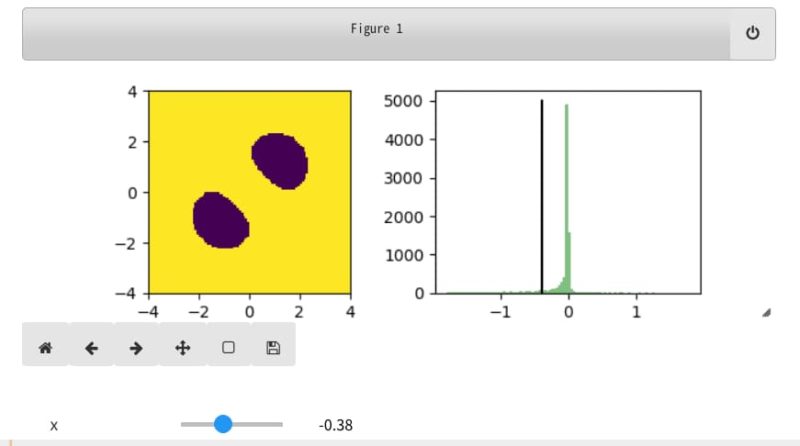
参考
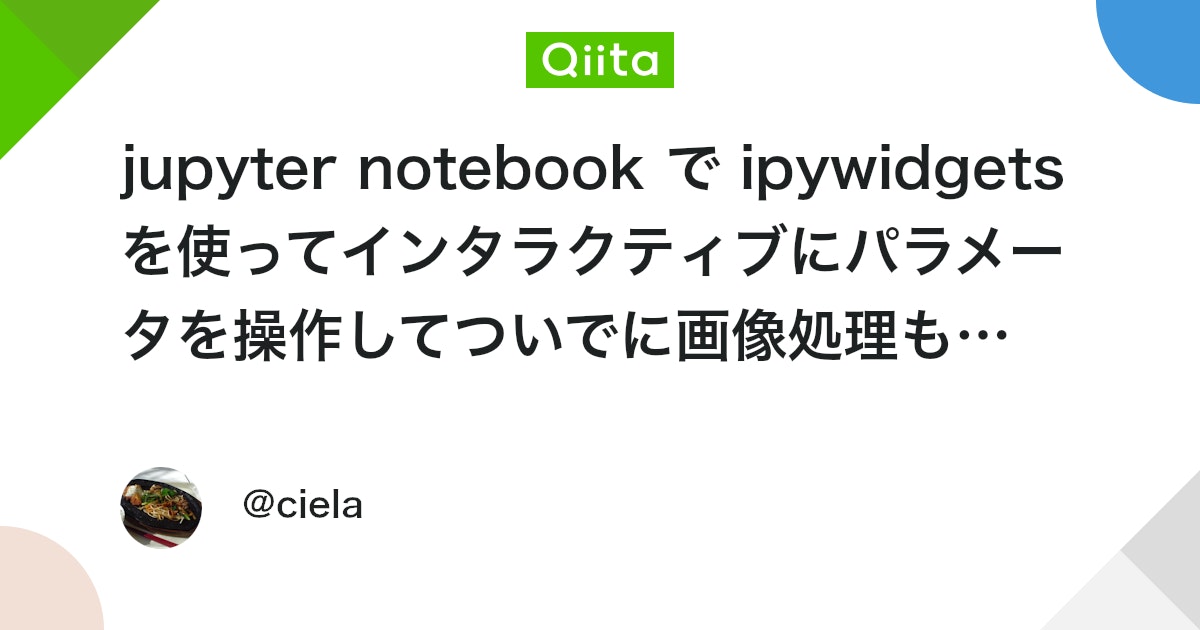
jupyter notebook で ipywidgets を使ってインタラクティブにパラメータを操作してついでに画像処理もしてみる - Qiita
matplotlib とかで jupyter notebook に関数のグラフをプロットするのはいいけど値を変更して試行錯誤するたびにいちいち Ctrl + Enter とかするのがちとめんどくさい。最近流行りのリアクティブ(もう古い?)的...
Using Interact — Jupyter Widgets 8.1.5 documentation
コメント