matplotlibでの画像の取扱方法
コード & 解説
モジュールのインポート
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
%matplotlib inline
from scipy import ndimage
import numpy as np
import matplotlib.image as mpimg
import matplotlib.pyplot as plt
plt.rcParams['savefig.dpi']=150
画像の読み込み
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
image = plt.imread('Lophophora.jpg')
plt.imread(‘ファイル名’)で、カレントディレクトリにある画像を読み込む。ここでは↓の画像を用いる。
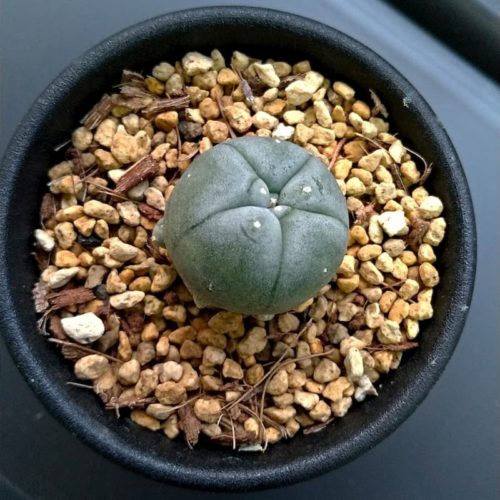
よみこんだ画像のサイズは、
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
image.shape
(800, 800, 3)
のようになっており、800×800の位置にRGBの色情報が入っているので(800,800,3)となる。
画像の表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#画像の表示
plt.imshow(image)
plt.imshow(image)で画像が表示される。
画像の保存
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#画像の保存
plt.imsave('ロフォフォラ.jpg', image)
plt.imsave(‘ロフォフォラ.jpg’, image)のように、imsave(‘ファイル名’, 保存したい画像ファイル)とすることで画像が保存できる。imsaveの場合、画像のみが保存され、枠線や目盛などは表示されない。
疑似カラーマップによる表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
img = image[:,:,0]
plt.imshow(img)
plt.savefig('ロフォフォラviridis.jpg')
RGBのRのデータのみをimshowに渡すと、疑似カラーマップによる表示となる。defaultはviridisであるため、下のようになる。
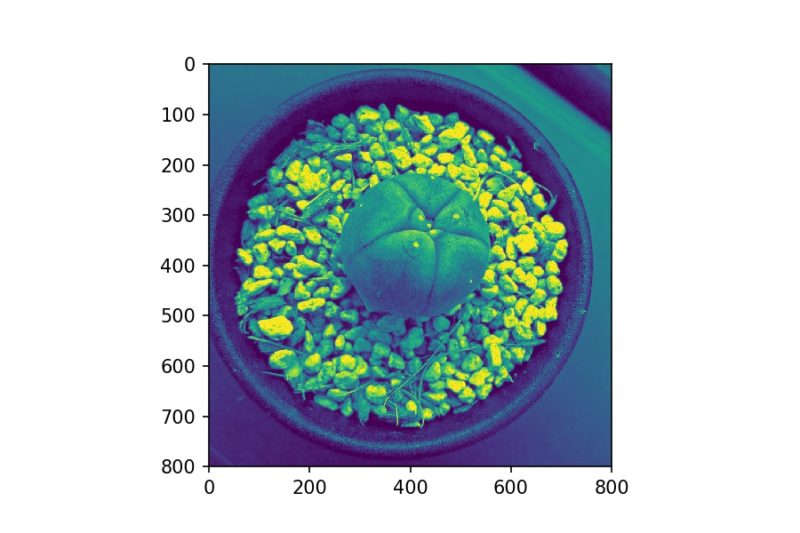
グレースケールによる表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
plt.imshow(img,cmap='Greys_r')
cmap=’Greys_r’とすることで、白黒画像となる。
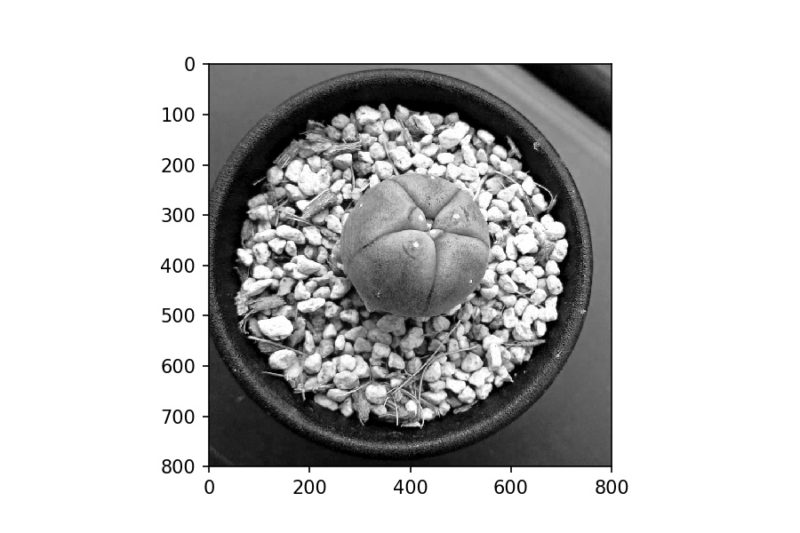
カラーバーの表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
plt.imshow(img,cmap='summer')
plt.colorbar()
plt.colorbar()でカラーバーが表示される。
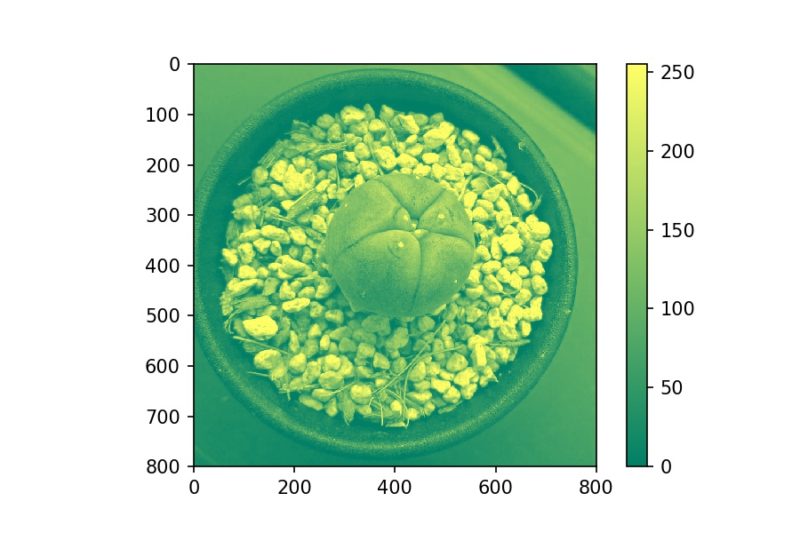
ヒストグラムの表示
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
plt.hist(img.ravel(), bins=256, range=(0.0, 256.0), fc='lightgreen')
plt.hist(img.ravel(), bins=256, range=(0.0, 256.0), fc=’lightgreen’)で画像のヒストグラムが作成できる。
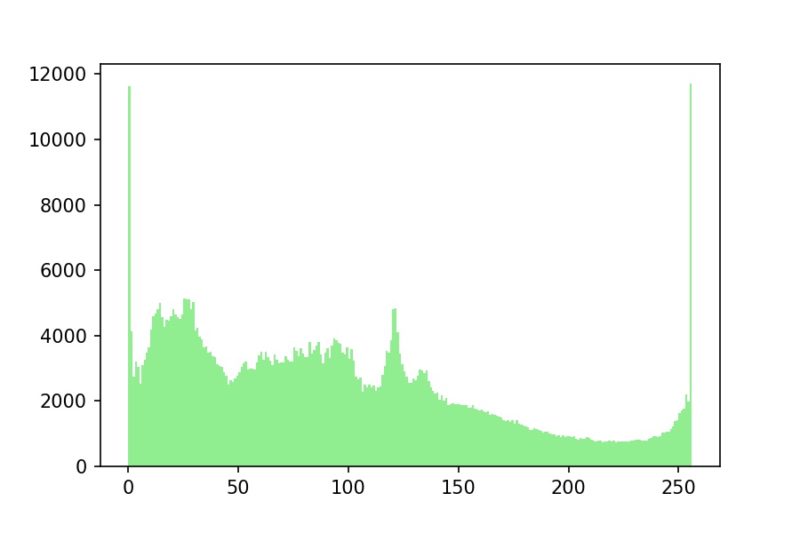
コントラストを高める
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from mpl_toolkits.axes_grid1 import ImageGrid
fig = plt.figure()
grid = ImageGrid(fig, 111, # similar to subplot(143)
nrows_ncols=(1, 2),
axes_pad=0.5,
label_mode="All",
share_all=True,
cbar_location="bottom",
cbar_mode="each",
cbar_size="8%",
cbar_pad="15%",
)
im1 = grid[0].imshow(img)
grid.cbar_axes[0].colorbar(im1)
im2 = grid[1].imshow(img, clim=(45, 180))
grid.cbar_axes[1].colorbar(im2)
grid[0].set_title('Before')
grid[1].set_title('After')
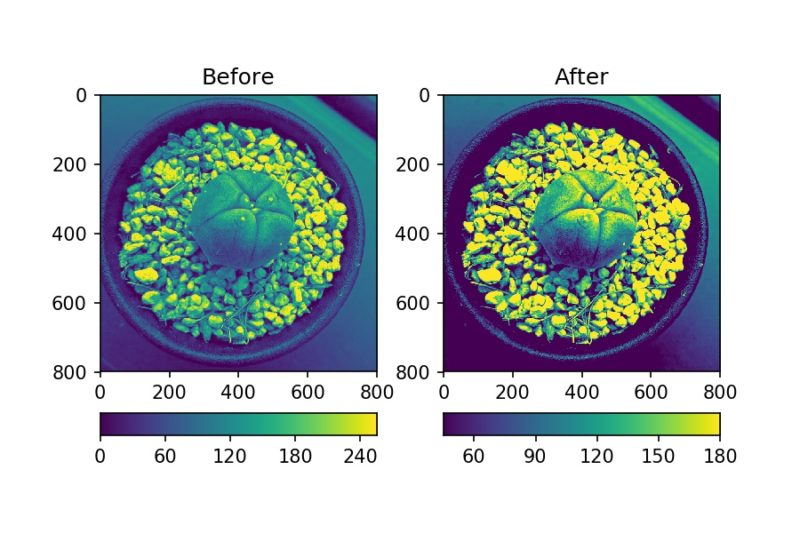
右の画像は、im2 = grid[1].imshow(img, clim=(45, 180))とヒストグラムの45から180の範囲でカラー設定をしているので、ハイコントラストな画像が得られる。
画像の補間
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from PIL import Image
img = Image.open('rophophora.jpg')
img.thumbnail((64, 64), Image.ANTIALIAS) # resizes image in-place
imgplot = plt.imshow(img)
from mpl_toolkits.axes_grid1 import ImageGrid
fig = plt.figure()
grid = ImageGrid(fig, 111, # similar to subplot(143)
nrows_ncols=(1, 3),
axes_pad=0.5,
label_mode="All",
share_all=True,
)
grid[0].imshow(img)
grid[1].imshow(img, interpolation="bilinear")
grid[2].imshow(img, interpolation="spline16")
grid[0].axis('off')
grid[1].axis('off')
grid[2].axis('off')
grid[0].set_title('None')
grid[1].set_title('bilinear')
grid[2].set_title('spline16')
x1, x2, y1, y2 = 16, 48, 44, 12
grid[0].set_xlim(x1, x2)
grid[0].set_ylim(y1, y2)
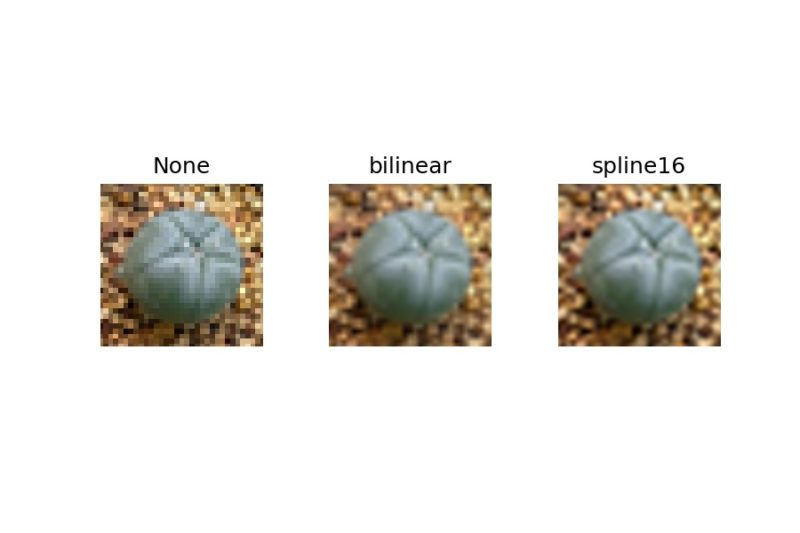
補間をわかりやすくするため、img.thumbnail((64, 64), Image.ANTIALIAS) で画像を粗くしている。
interpolation=”bilinear”のように、補間方法を指定することで滑らかに保管された画像が得られる。補間方法は、15種類ほどある。
Interpolations for imshow — Matplotlib 3.10.1 documentation
参考
https://matplotlib.org/tutorials/introductory/images.html#sphx-glr-tutorials-introductory-images-py
Interpolations for imshow — Matplotlib 3.10.1 documentation

ロフォフォラ - Wikipedia
コメント
[…] [matplotlibの使い方] 24. 画像 2019.03.182019.11.03 WindowsオフラインパソコンにcondaでPython環境構築 2019.06.072019.10.11 [SciPy] 1. Scipyのcurve_fitで最小2乗法近似、決定係数R2も求める […]
[…] [matplotlibの使い方] 24. 画像matplotlibでの画像の取扱方法sabopy.com2019.03.18 […]