はじめに
matplotlibのtriplotでデータポイントのドロネー三角形分割を表示する方法について説明する。
コード
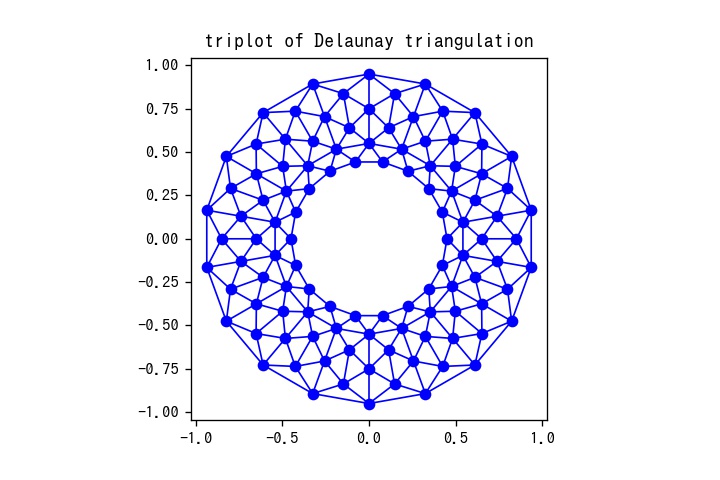
解説
モジュールのインポート
データの生成
np.repeat(angles[…, np.newaxis], n_radii, axis=1)でanglesをn_radii回、列方向に繰り替えした配列が得られるので、形状は(18, 6)となる。
angles[:, 1::2] += np.pi / n_angles で1列目のデータから一個飛ばしで要素にnp.pi / n_anglesを加える。
ドロネー三角形分割の生成
tri.Triangulation(x, y)でドロネー三角形分割が生成される。
ドロネー三角形分割のマスク処理
hypotは2乗和の平方根を返すのでnp.hypot(x[triang.triangles].mean(axis=1), y[triang.triangles].mean(axis=1)) < min_radiusで原点からの距離がmin_radiusより小さいところがTrueとなる。これをmaskするのでTrueの部分で点と線が表示されないこととなる。
triplotでドロネー三角形分割を表示
データの一部分を回転させなかったときの図
angles[:, 1::2] += np.pi / n_angles #swirl data
を実行しなかったときの図は下のようになる。
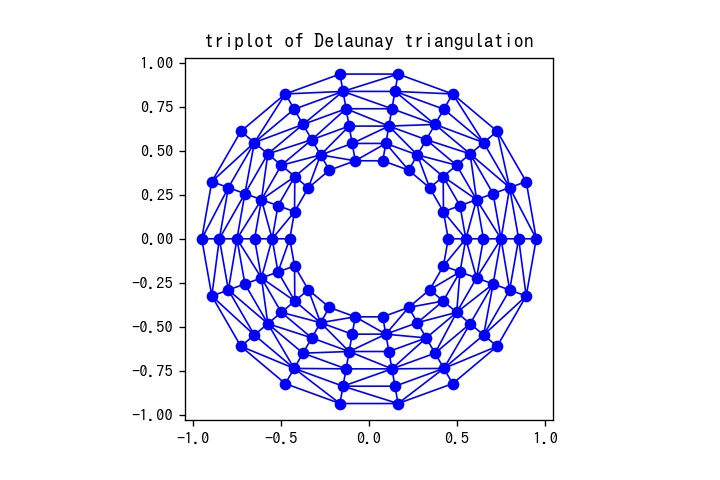
参考
Triplot Demo — Matplotlib 3.1.2 documentation
matplotlib.pyplot.triplot — Matplotlib 3.1.2 documentation
numpy.hypot — NumPy v2.1 Manual
コメント