はじめに
散布図を作成できるmatplotlibのplt.scatterで点の色を変えて表示する場合、その色が示すものを説明する必要がある。そこで離散的なカラーバーとともに散布図を表示する方法について説明する。
コード
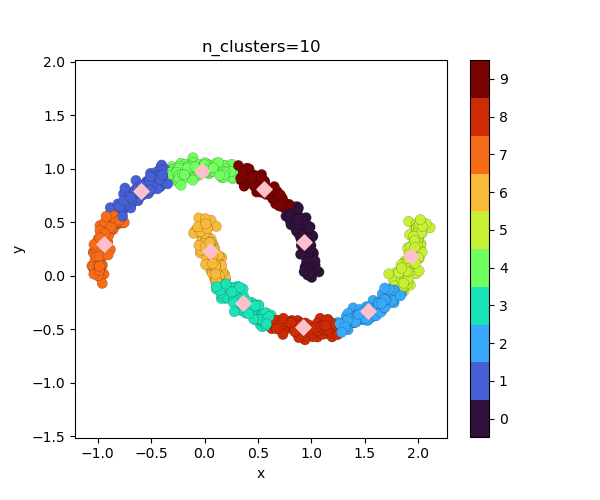
解説
モジュールのインポートなど
バージョン
データの生成
sklearnのdatasets.make_moons
で三日月状の分布を示すクラスタリング、分類用のデータを作成する。詳細は下記記事で解説した。Xにデータの座標が入り、yにラベルの値が入る。
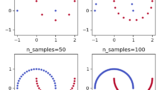
[scikit-learn] 4. make_moonsによる三日月状データの生成
sklearnのdatasets. make_moonsで三日月状の分布を示すクラスタリング、分類用のデータを作成することができる。ここでは各種パラメータが生成データに及ぼす影響について説明する。
図示すると以下のようになる。
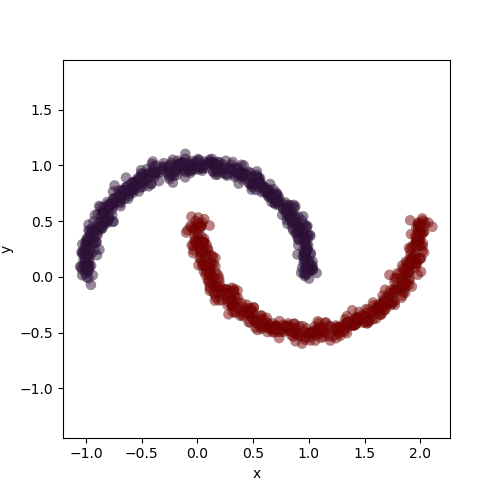
KMeansによるクラスタリング
クラスター数を10として、k平均法によるクラスタリングを行う。y_10_はそれぞれのデータをクラスタリングした結果(ラベル)となる。
離散的カラーバーのための準備
レベルを設定する。値がレベルの範囲内におさまるように全体から-.0001している。
BoundaryNormでレベルに基づいて、カラーマップインデックスを生成する。
離散的カラーバーつき散布図の表示
ax.scatter()でnorm=normとする。
各クラスター中心はax.scatter(fit_10.cluster_centers_[:,0],fit_10.cluster_centers_[:,1])で表示した。
カラーバーはplt.colorbar(p,ticks=np.arange(10)+.5)として、目盛りの値が各カラーの真ん中に来るように+0.5とした。目盛りの値はcbar.ax.set_yticklabels()で設定できる。
参考
matplotlib.pyplot.colorbar — Matplotlib 3.3.4 documentation

KMeans
Gallery examples: Release Highlights for scikit-learn 1.1 Release Highlights for scikit-learn 0.23 A demo of K-Means clu...
コメント