はじめに
skimage.filters の windowを画像のフーリエ変換に適用する方法について説明する。
解説
モジュールのインポートなど
バージョン
画像の読み込み
アストロフィツム属の兜丸の写真を用いる。rgb2grayでグレースケール画像に変換する。
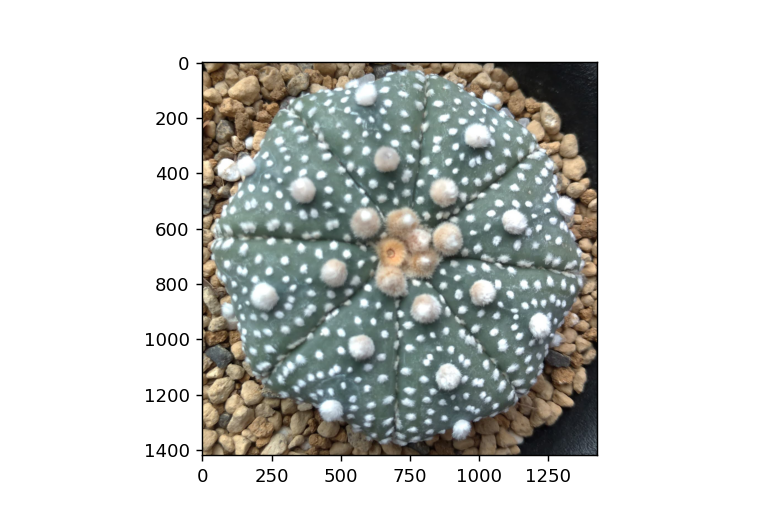
窓関数をかける
gaussianフィルターでノイズを滑らかにした後に窓関数をかける。利用できるのはscipyの窓関数であり、ここでは’hann’を用いる。そのほかに使用できる窓関数は下記ページに載っている。
Window functions (scipy.signal.windows) — SciPy v1.15.2 Manual
フーリエ変換
scipyのfft2でフーリエ変換をする。
結果の表示
左上が元画像、右上が窓関数をかけた画像で、左下が元画像のフーリエ変換の結果で、右下が窓関数をかけた画像のフーリエ変換の結果となる。窓関数の効果により、十字模様が消えて、鮮明な結果が得られている。
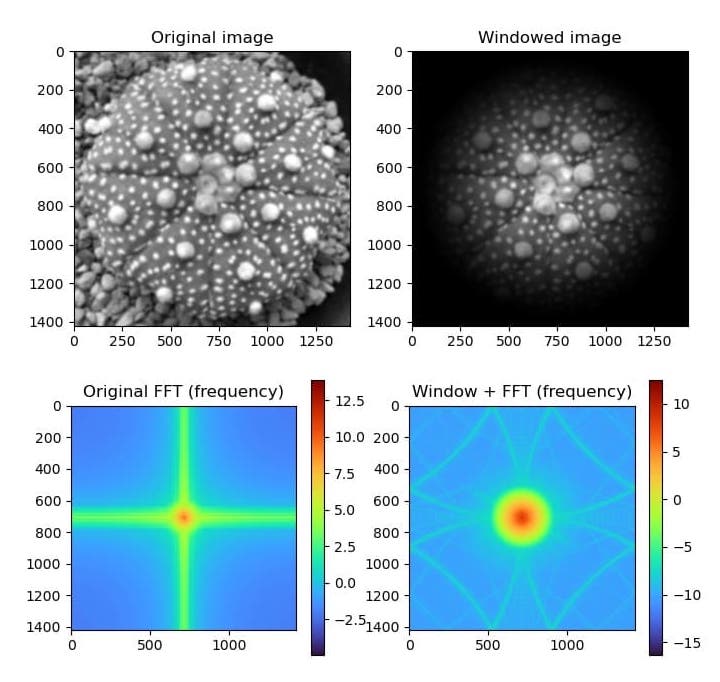
縦に線分析
縦方向のラインプロファイルは以下のようになる。
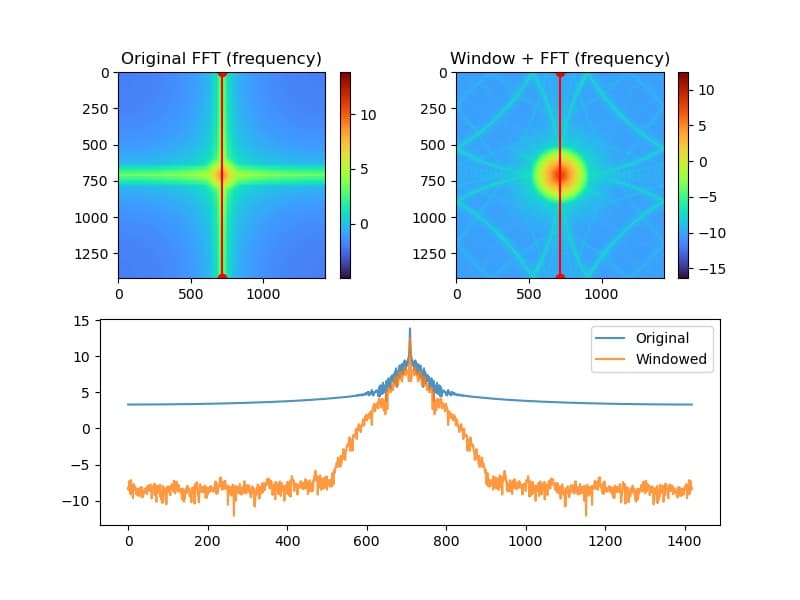
横に線分析
横方向のラインプロファイルは以下のようになる。
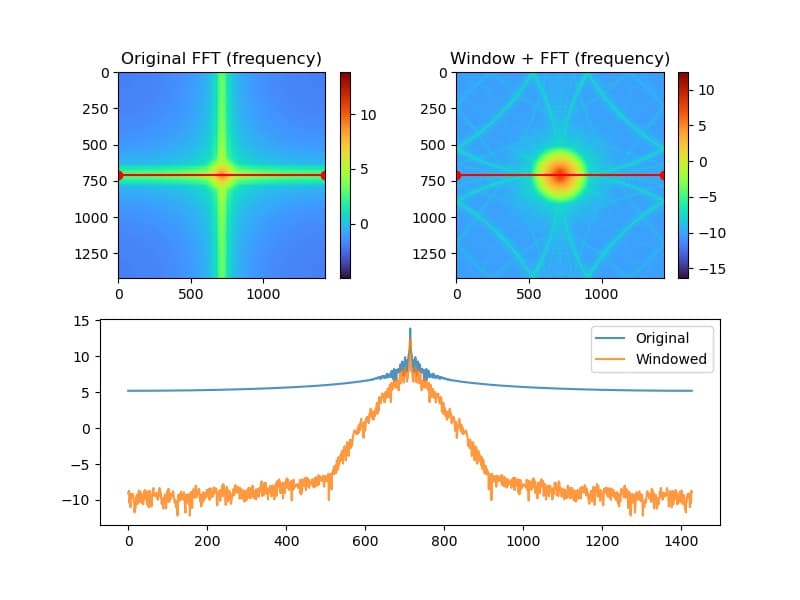
参考
Using window functions with images — skimage 0.26.0rc0.dev0 documentation
fft2 — SciPy v1.15.2 Manual
コメント