はじめに
matplotlib FuncAnimationによる3Dグラフの縦回転アニメーションについて説明する。
コード
解説
モジュールのインポート
グラフの設定
ピラミッドの作成
ピラミッドはボクセルで↓と同様に作成。
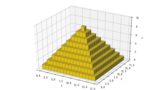
[matplotlib 3D] 46.ボクセルピラミッド(Voxel Pyramid)
matplotlib mplot3dでvoxelによるピラミッド
アニメーションの設定
視点変更するための配列の生成
3Dグラフの高さ方向の視点elevにおいて、180と-180はおなじなので、endpoint = Falseとすることで180を配列にいれないようにした。np.concatenate()で配列を連結する。配列の大きさは101とした。
アニメーションの設定
アニメーションの初期設定でグラフのアスペクト比とz軸範囲を調整する。
ax.view_init()で視点を変更できる。
azim=60と固定で、elev=rotate_elev[i]でelevが変化するアニメーションとする。
アニメーションの表示
HTML(ani.to_html5_video())とすればjupyter notebook上にアニメーションを表示できる。
参考
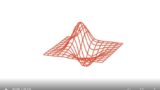
[matplotlib 3D] 14. 3Dグラフの回転アニメーション
matplotlib mplot3d のグラフの回転アニメーション
コメント