はじめに
sklearnのdatasets.make_regression
で回帰問題用のランダムなデータを作成することができる。ここでは各種パラメータが生成するデータに及ぼす影響について説明する。
解説
モジュールのインポートなど
バージョン
n_samples
n_samplesを変化させることでサンプル数を変えることができる。
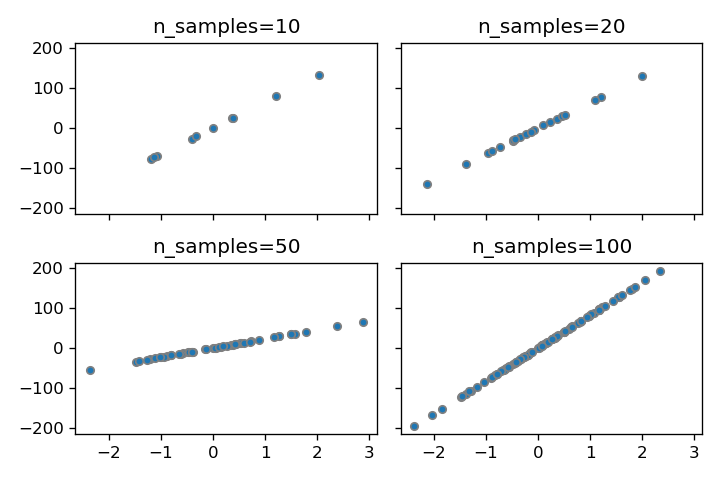
n_features
データの列数を変えることができる。
n_informative
線形モデルに適合するデータ(列)の数を設定できる。1と2とした場合は以下のようになる。2は左上と右下が線形モデルに合うように思える。
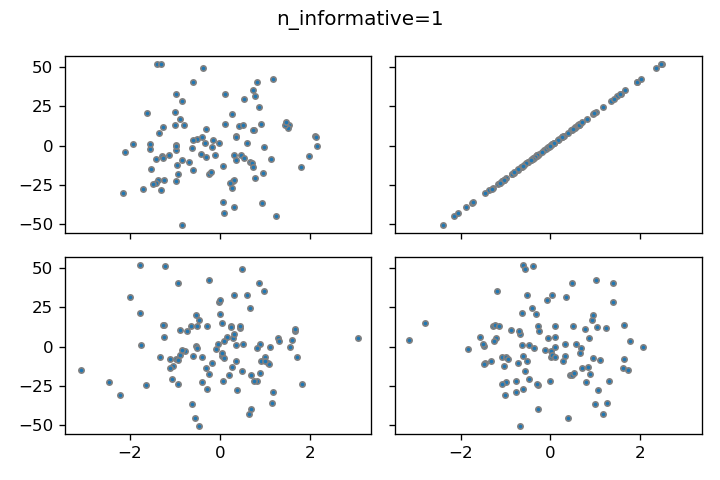
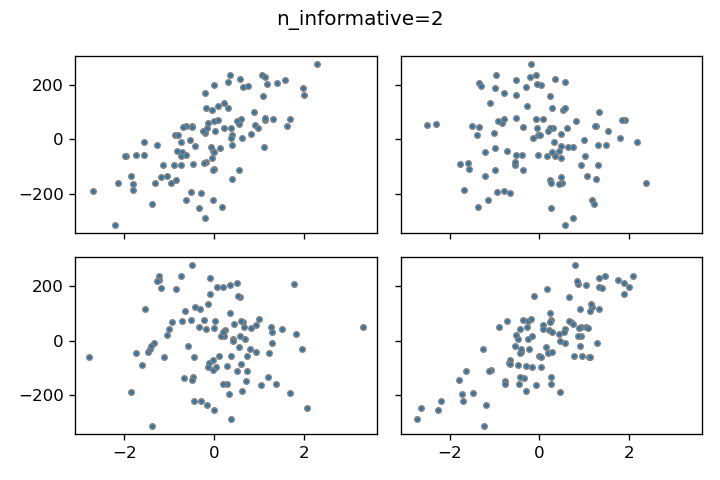
n_targets
n_targetsは出力値Yの数となる。n_targets=4として異なるYとXをプロットすると以下のようになる。
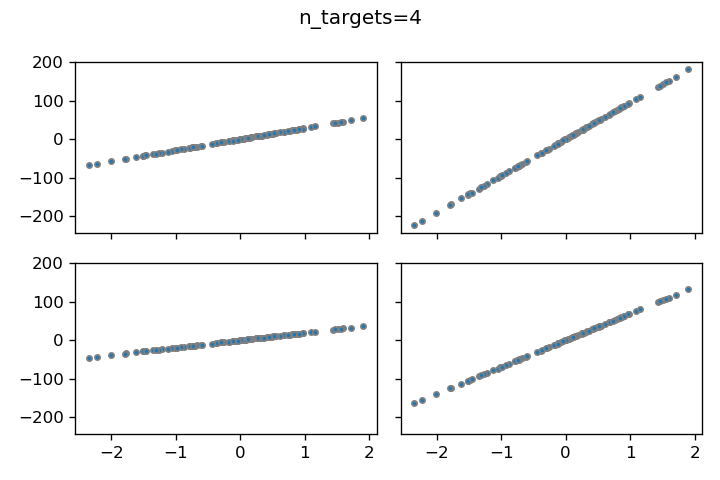
bias
biasは切片の値となる。
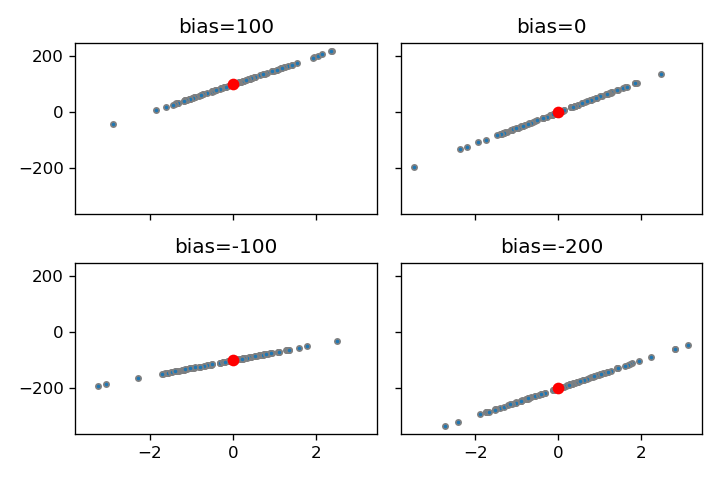
noise
noiseでばらつきを付与できる。
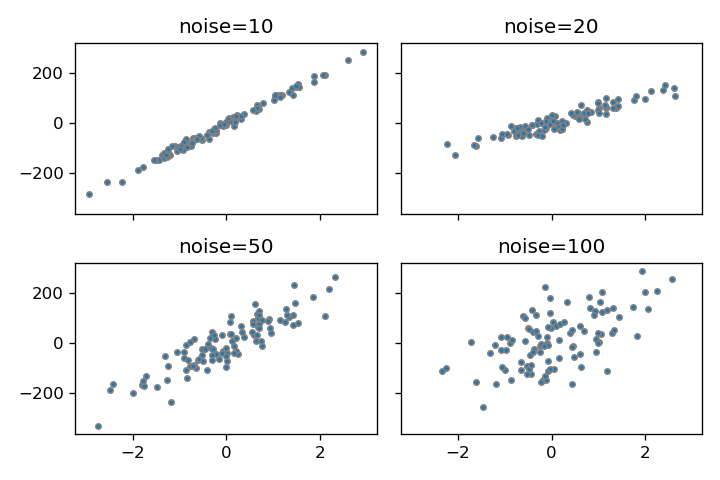
random_state
random_stateを変えることで再現可能な乱数を生成することができる。
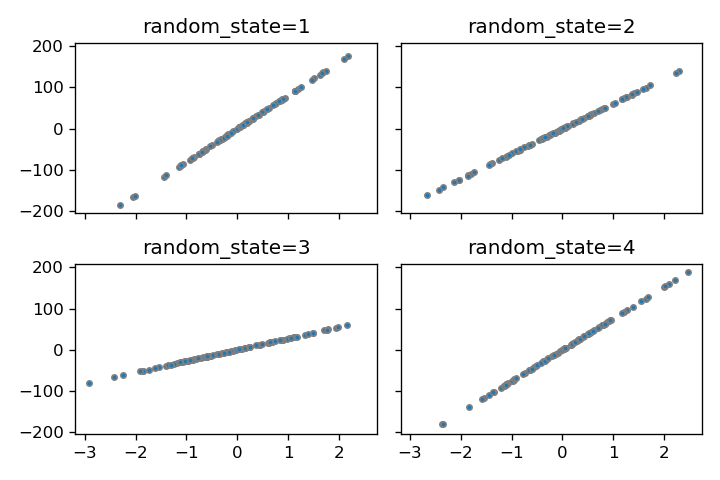
coef
coefをTrueとすることで線形モデルの係数を得ることができる。デフォルトはFalse.
n_features=4とすれば4つのcoefが得られる。
参考

make_regression
Gallery examples: Release Highlights for scikit-learn 1.4 Release Highlights for scikit-learn 0.23 Prediction Latency Co...
コメント