はじめに
ここでは、skimage restoration unwrap_phaseによりラッピングした画像をアンラッピングする例について説明する。
コード
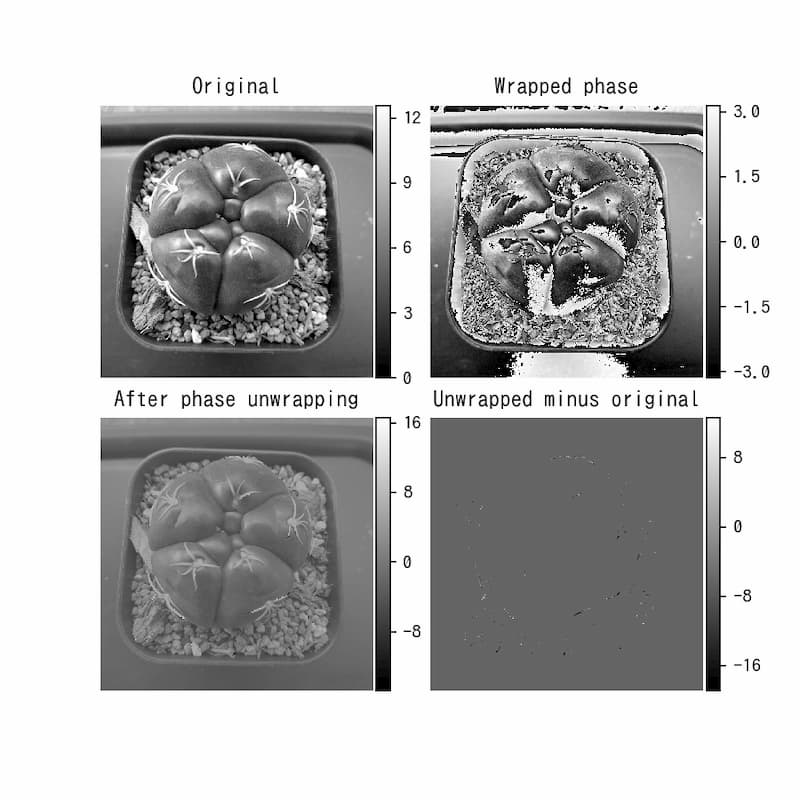
解説
モジュールのインポート
画像データの読み込みとグレースケール化
ギムノカリキュウム・海王丸の画像を読み込む。
データ形式を0-1のfloat型にした後にrgb2grayによりグレースケールにする。
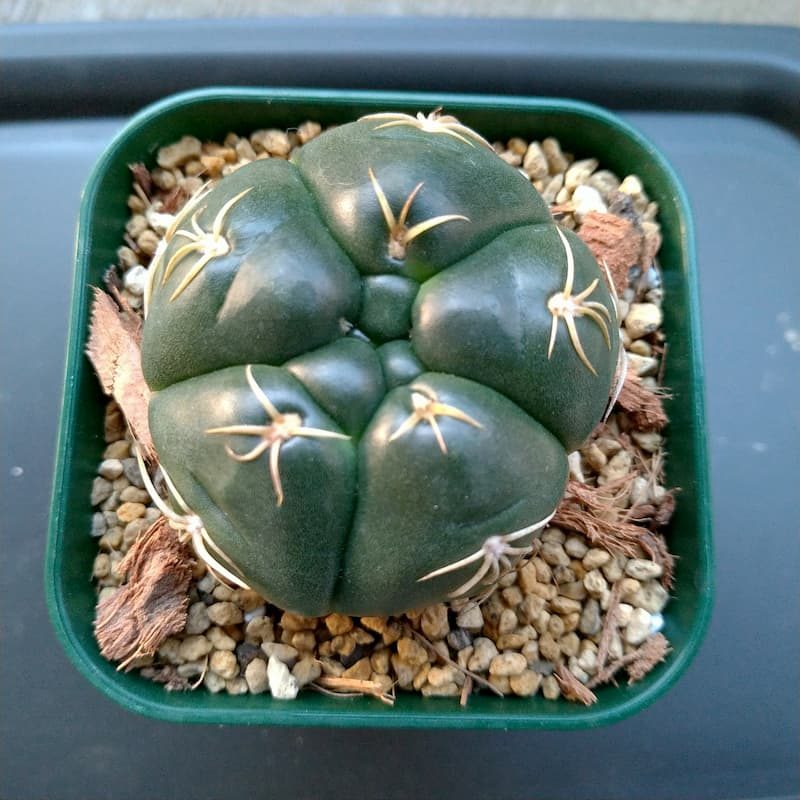
画像のデータ範囲をリスケール
exposure.rescale_intensity()によりデータの範囲を(0, 4 * np.pi)にリスケールする。
rescale_intensityについては下記で解説した。
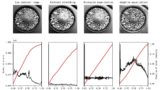
[scikit-image] 8. 各種均等化法によるコントラストの補正
skimage.exposureのrescale_intensity, equalize_histとequalize_adapthistによる画像のコントラストの補正
位相ラッピング像の生成
1j * imageにより画像を複素数にする。それの指数をnp.exp()でとることでオイラーの公式が適用できて、np.angle()で偏角をとることで(-π, π)でラッピングされたデータを得ることができる。
位相アンラッピング像の生成
画像の表示
Imagegrid により画像を表示した。
cbar_mode=”each”などを記述しないとカラーバーは表示されない。
cbar_pad=0.03は画像とカラーバーの間隔となる。
Imagegridでカラーバーを表示するには、grid.cbar_axes[3].colorbar(im3)のようにする。
コードをダウンロード(.pyファイル) コードをダウンロード(.ipynbファイル)参考
Phase Unwrapping — skimage 0.24.0 documentation

オイラーの公式 - Wikipedia
位相アンラッピング(Phase unwrapping)
1D Phase Unwappingをサクッと行う 1.元データの作成 まず元データを作成。適当なデータを作る。 In : from numpy imp...
コメント